2023/03/29
InheritedWidgetやBLOC、Providerなどの状態管理の基礎を勉強中。
コードは微修正程度。
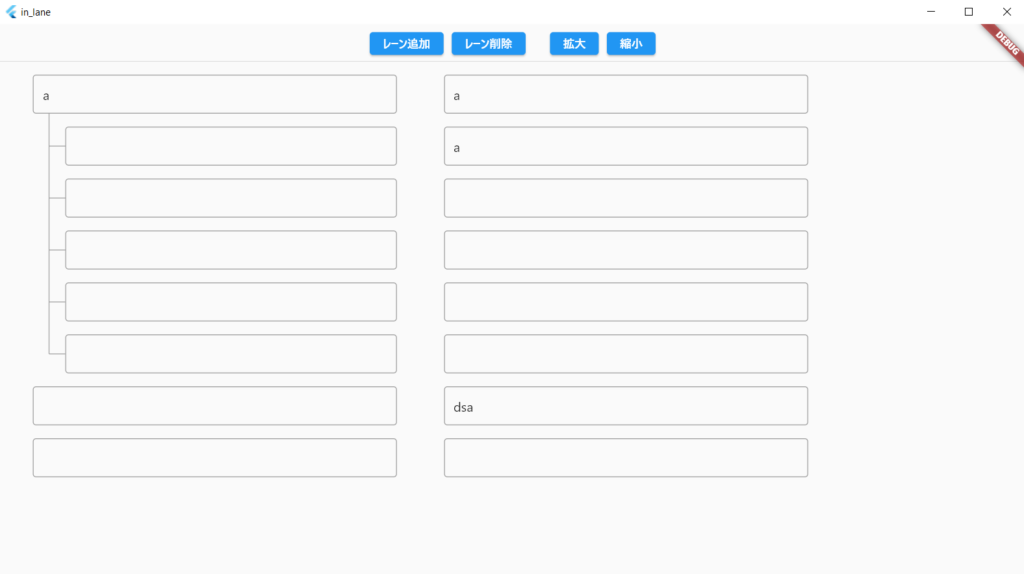
2023/04/01
ファイルへの保存・読込機能とタイトル欄を付けた。
基礎を勉強したおかげでFlutterに慣れてきた。
ちなみにキャプチャ内にある★3キャラというのは、東方アルカディアレコードというゲームを指している。
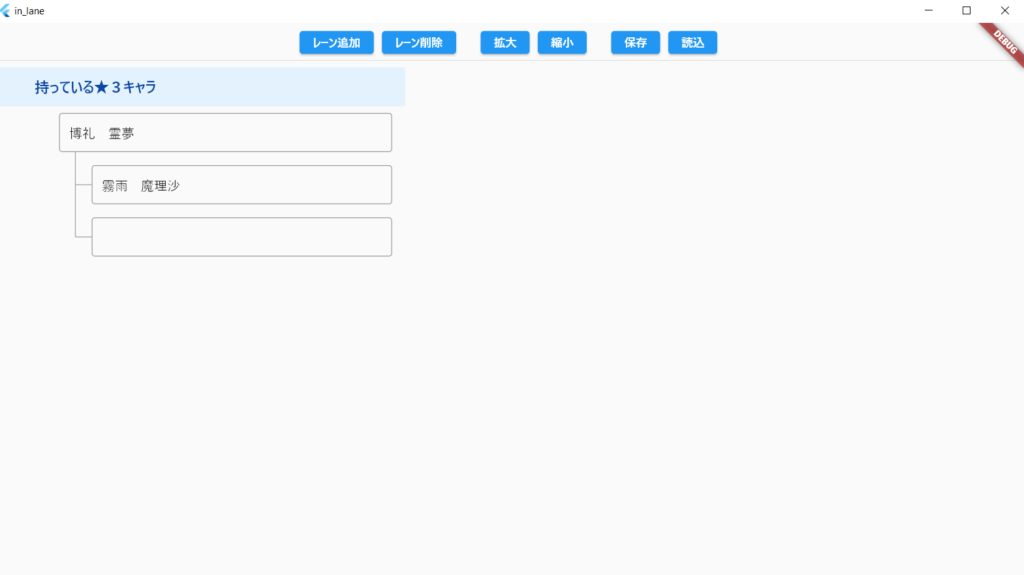
main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'indent_line_painter.dart';
import 'dart:math';
import 'dart:convert'; //JSON形式のデータを扱う
import 'package:path_provider/path_provider.dart';
import 'dart:io';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
body: SafeArea(child: CanvasZone()),
),
);
}
}
class CanvasZone extends StatefulWidget {
@override
State<CanvasZone> createState() => _CanvasZoneState();
}
class _CanvasZoneState extends State<CanvasZone> {
double _scale = 1.0;
List<Lane> lanes = [];
@override
void initState() {
super.initState();
addNewLane();
}
void addNewLane() {
setState(() {
lanes.add(Lane(
key: GlobalKey<_LaneState>(),
index: lanes.length,
ideas: [Idea(key: GlobalKey<_IdeaState>(), index: 0)]));
});
}
void removeLane() {
setState(() {
if (lanes.length > 1) {
lanes.removeLast();
}
});
}
void focusNextLane(int targetLaneIndex, int targetIdeaIndex) {
if (targetLaneIndex < 0 || targetIdeaIndex < 0) return;
if (targetLaneIndex == lanes.length) {
addNewLane();
}
if (targetLaneIndex < lanes.length) {
for (int i = lanes[targetLaneIndex].ideas.length - 1;
i < targetIdeaIndex;
i++) {
if(lanes[targetLaneIndex].key.currentState == null) {
setState(() {
lanes[targetLaneIndex].ideas.add(Idea(key: GlobalKey<_IdeaState>(), index: i + 1));
for (int j = 0; j < lanes[targetLaneIndex].ideas.length; j++) {
lanes[targetLaneIndex].ideas[j].index = j;
}
});
} else {
lanes[targetLaneIndex].key.currentState?.addNewIdea(i);
}
};
lanes[targetLaneIndex]
.ideas[targetIdeaIndex]
.key
.currentState
?._textFocusNode
.requestFocus();
}
}
void scaleUp() {
setState(() {
_scale += 0.1;
});
}
void scaleDown() {
setState(() {
_scale = max(_scale - 0.1, 0.1);
});
}
Future<File> get _localFile async {
final directory = await getApplicationDocumentsDirectory();
return File('${directory.path}/lanes_and_ideas.json');
}
Future<void> loadData() async {
final file = await _localFile;
if (await file.exists()) {
String jsonString = await file.readAsString();
Map<String, dynamic> jsonMap = jsonDecode(jsonString);
List<Lane> loadedLanes = [];
for (var laneJson in jsonMap['lanes']) {
List<Idea> loadedIdeas = [];
for (var ideaJson in laneJson['ideas']) {
loadedIdeas.add(Idea(
key: GlobalKey<_IdeaState>(),
index: ideaJson['index'],
indentLevel: ideaJson['indentLevel'],
));
}
loadedLanes.add(Lane(
key: GlobalKey<_LaneState>(),
index: laneJson['index'],
ideas: loadedIdeas,
));
}
setState(() {
lanes = loadedLanes;
for (int i = 0; i < lanes.length; i++) {
for (int j = 0; j < lanes[i].ideas.length; j++) {
lanes[i].ideas[j].content =
jsonMap['lanes'][i]['ideas'][j]['content'];
}
}
});
} else {
print('No lanes and ideas to load from JSON');
}
}
Future<void> saveData() async {
List<Map<String, dynamic>> lanesJson = [];
for (var lane in lanes) {
List<Map<String, dynamic>> ideasJson = [];
for (var idea in lane.ideas) {
ideasJson.add({
'index': idea.index,
'indentLevel': idea.indentLevel,
'content': idea.key.currentState?._textController.text ?? '',
});
}
lanesJson.add({
'index': lane.index,
'ideas': ideasJson,
});
}
String jsonString = jsonEncode({'lanes': lanesJson});
final file = await _localFile;
await file.writeAsString(jsonString);
}
@override
Widget build(BuildContext context) {
return CanvasZoneInheritedWidget(
canvasZoneState: this,
child: Column(
children: [
Align(
alignment: Alignment.topCenter,
child: Container(
margin: EdgeInsets.only(top: 10),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: addNewLane,
child: Text("レーン追加"),
),
SizedBox(width: 10),
ElevatedButton(
onPressed: removeLane,
child: Text("レーン削除"),
),
SizedBox(width: 30),
ElevatedButton(
onPressed: scaleUp,
child: Text("拡大"),
),
SizedBox(width: 10),
ElevatedButton(
onPressed: scaleDown,
child: Text("縮小"),
),
SizedBox(width: 30),
ElevatedButton(
onPressed: saveData,
child: Text("保存"),
),
SizedBox(width: 10),
ElevatedButton(
onPressed: loadData,
child: Text("読込"),
),
],
),
),
),
const Divider(),
Expanded(
child: Transform.scale(
scale: _scale,
alignment: Alignment.topLeft,
child: ListView.builder(
scrollDirection: Axis.horizontal,
itemCount: lanes.length,
itemBuilder: (context, index) {
return Container(
width: MediaQuery.of(context).size.width * 0.4,
child: lanes[index],
);
},
),
),
),
],
),
);
}
}
class CanvasZoneInheritedWidget extends InheritedWidget {
final _CanvasZoneState canvasZoneState;
CanvasZoneInheritedWidget(
{required this.canvasZoneState, required Widget child})
: super(child: child);
@override
bool updateShouldNotify(CanvasZoneInheritedWidget oldWidget) => true;
static _CanvasZoneState of(BuildContext context) {
return context
.dependOnInheritedWidgetOfExactType<CanvasZoneInheritedWidget>()!
.canvasZoneState;
}
}
class Lane extends StatefulWidget {
int index;
final GlobalKey<_LaneState> key;
List<Idea> ideas;
final double indentWidth=40; //インデントの下げ幅
Lane({
required this.key,
required this.index,
required this.ideas
}) : super(key: ValueKey('Lane'+key.toString()));
@override
_LaneState createState() => _LaneState();
}
class _LaneState extends State<Lane> {
List<Idea> get ideas => widget.ideas;
TextEditingController _titleController = TextEditingController();
FocusNode _titleFocusNode = FocusNode();
void addNewIdea(int currentIndex) {
setState(() {
int currentIndentLevel =
ideas[currentIndex].indentLevel ?? 0;
ideas.insert(
currentIndex + 1,
Idea(
key: GlobalKey<_IdeaState>(),
index: currentIndex + 1,
textFieldHeight: 48.0,
indentLevel: currentIndentLevel,
),
);
for (int i = currentIndex + 2; i < ideas.length; i++) {
ideas[i].index = i;
}
});
}
void moveIdea(int currentIndex, int direction) {
if (currentIndex + direction >= 0 &&
currentIndex + direction < ideas.length) {
setState(() {
Idea temp = ideas[currentIndex];
ideas[currentIndex] = ideas[currentIndex + direction];
ideas[currentIndex + direction] = temp;
ideas[currentIndex].key.currentState?.updateIndex(currentIndex);
ideas[currentIndex + direction]
.key
.currentState
?.updateIndex(currentIndex + direction);
});
}
}
void deleteIdea(int currentIndex) {
if (ideas.length > 1) {
setState(() {
ideas.removeAt(currentIndex);
for (int i = currentIndex; i < ideas.length; i++) {
ideas[i].index = i;
}
if (currentIndex > 0) {
ideas[currentIndex - 1]
.key
.currentState
?._textFocusNode
.requestFocus();
} else {
ideas[currentIndex].key.currentState?._textFocusNode.requestFocus();
}
});
}
}
void focusAdjacentIdea(int currentIndex, int direction) {
if (currentIndex + direction >= 0 &&
currentIndex + direction < ideas.length) {
ideas[currentIndex + direction]
.key
.currentState
?._textFocusNode
.requestFocus();
}
}
void redrawAffectedIdeas(int startIndex, int endIndex) {
if (startIndex < 0 || endIndex >= ideas.length) return;
setState(() {
for (int i = startIndex; i <= endIndex; i++) { //再描画を促す
//ideas[i].key.currentState?.indentLevel = widget.key.currentState?.indentLevel ?? indentLevel;
}
});
}
@override
Widget build(BuildContext context) {
return LaneInheritedWidget(
laneState: this,
child: Column(
children: [
Container(
color: Colors.blue[50],
padding: EdgeInsets.symmetric(horizontal: widget.indentWidth),
child: TextField(
controller: _titleController,
focusNode: _titleFocusNode,
decoration: InputDecoration(
border: InputBorder.none,
disabledBorder: OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 2.0),
),
// labelText: '',
fillColor: Colors.blue[50],
filled: true,
),
style: TextStyle(fontSize: 18.0, fontWeight: FontWeight.bold, color: Colors.blue[900]),
keyboardType: TextInputType.text,
textInputAction: TextInputAction.done,
onSubmitted: (value) {
_titleFocusNode.unfocus();
},
),
),
Expanded(
child: ListView.builder(
itemCount: ideas.length,
itemBuilder: (context, index) {
return ideas[index];
},
),
),
]
),
);
}
}
class LaneInheritedWidget extends InheritedWidget {
final _LaneState laneState;
LaneInheritedWidget({required this.laneState, required Widget child})
: super(child: child);
@override
bool updateShouldNotify(LaneInheritedWidget oldWidget) => true;
static _LaneState of(BuildContext context) {
return context
.dependOnInheritedWidgetOfExactType<LaneInheritedWidget>()!
.laneState;
}
}
class Idea extends StatefulWidget {
int index;
int indentLevel;
final GlobalKey<_IdeaState> key;
final double boxSpacing; //他のエディタとの間隔
final double textFieldHeight; //テキスト欄の高さ
String content;
Idea({
required this.key,
required this.index,
this.boxSpacing = 8.0,
this.textFieldHeight = 48.0, //デフォルトは48
this.indentLevel = 0,
this.content = '',
}) : super(key: ValueKey('Idea'+key.toString()));
@override
_IdeaState createState() => _IdeaState();
}
class _IdeaState extends State<Idea> {
TextEditingController _textController = TextEditingController();
FocusNode _textFocusNode = FocusNode();
void updateIndex(int newIndex) {
setState(() {
widget.index = newIndex;
});
}
void updateIndentLevel() {
setState(() {
});
}
// インデントの線を描画する
void _indentLinePaint(Canvas canvas, Size size) {
indentLinePaint(canvas, size, context, widget.index, widget.indentLevel,
widget.textFieldHeight, widget.boxSpacing, LaneInheritedWidget.of(context).widget.indentWidth);
}
String toJson() {
Map<String, dynamic> data = {
'index': widget.index,
'indentLevel': widget.indentLevel ?? 0,
'text': _textController.text ?? "",
};
return json.encode(data);
}
@override
void initState() {
super.initState();
_textController.text = widget.content;
WidgetsBinding.instance.addPostFrameCallback((_) {
FocusScope.of(context).requestFocus(_textFocusNode);
});
}
@override
Widget build(BuildContext context) {
return Focus(
onKey: (FocusNode node, RawKeyEvent event) {
if (event.runtimeType == RawKeyDownEvent) {
if (event.logicalKey == LogicalKeyboardKey.capsLock) {
// CapsLockキーを無視する条件を追加
return KeyEventResult.ignored;
} else if (event.isControlPressed) { //コントロールキー
bool needRepaint = false;
if (event.logicalKey == LogicalKeyboardKey.arrowUp) {
LaneInheritedWidget.of(context).moveIdea(widget.index, -1);
needRepaint = true;
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) {
LaneInheritedWidget.of(context).moveIdea(widget.index, 1);
needRepaint = true;
}
if (needRepaint) {
findAndRepaintAffectedIdeas(context, widget.index, widget.indentLevel,
widget.index, widget.index + 1 /*Ctrlキーの場合、1つ下からチェックが必要*/);
}
} else if (event.logicalKey == LogicalKeyboardKey.arrowUp) { //カーソルキー ↑
LaneInheritedWidget.of(context)
.focusAdjacentIdea(widget.index, -1);
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) {
if (widget.index ==
LaneInheritedWidget.of(context).ideas.length - 1) {
LaneInheritedWidget.of(context).addNewIdea(widget.index);
} else {
LaneInheritedWidget.of(context)
.focusAdjacentIdea(widget.index, 1);
}
} else if (event.logicalKey == LogicalKeyboardKey.arrowRight && //カーソルキー →
_textController.selection.baseOffset ==
_textController.text.length) {
int laneIndex = CanvasZoneInheritedWidget.of(context)
.lanes
.indexOf(LaneInheritedWidget.of(context).widget);
CanvasZoneInheritedWidget.of(context)
.focusNextLane(laneIndex + 1, widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft &&
_textController.selection.baseOffset == 0) {
int laneIndex = CanvasZoneInheritedWidget.of(context)
.lanes
.indexOf(LaneInheritedWidget.of(context).widget);
CanvasZoneInheritedWidget.of(context)
.focusNextLane(laneIndex - 1, widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.tab) { //TABキー
if (event.isShiftPressed) {
setState(() {
widget.indentLevel = max(0, widget.indentLevel - 1);
});
} else {
setState(() {
widget.indentLevel += 1;
});
}
findAndRepaintAffectedIdeas(context, widget.index, widget.indentLevel,
widget.index, widget.index);
return KeyEventResult.handled; //デフォルトのタブの挙動を無効化して、フォーカスの移動を防ぐ
} else if ((event.logicalKey == LogicalKeyboardKey.delete ||
event.logicalKey == LogicalKeyboardKey.backspace) &&
_textController.text.isEmpty) {
LaneInheritedWidget.of(context).deleteIdea(widget.index);
}
}
return KeyEventResult.ignored;
},
child: RawKeyboardListener(
focusNode: FocusNode(),
child: Container(
margin: EdgeInsets.symmetric(
horizontal: 16, vertical: widget.boxSpacing),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const SizedBox(
width: 26,
child: DecoratedBox(
decoration: BoxDecoration(color: Colors.red))),
Expanded(
flex: 4,
child: Row(children: [
CustomPaint(
painter: _CustomPainter(_indentLinePaint),
child: Container(width: LaneInheritedWidget.of(context).widget.indentWidth * widget.indentLevel),
),
Expanded(
child: Container(
height: widget.textFieldHeight,
child: TextField(
controller: _textController,
focusNode: _textFocusNode,
maxLines: 1,
decoration: const InputDecoration(
border: OutlineInputBorder(),
),
keyboardType: TextInputType.multiline,
textInputAction: TextInputAction.done,
onSubmitted: (value) {
_textFocusNode.unfocus();
LaneInheritedWidget.of(context)
.addNewIdea(widget.index);
},
),
),
),
]),
),
],
),
),
));
}
}
class _CustomPainter extends CustomPainter {
final void Function(Canvas, Size) _paint;
_CustomPainter(this._paint);
@override
void paint(Canvas canvas, Size size) {
_paint(canvas, size);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) => false;
}
※本記事は、過去の記録(メモ)をもとに、他の方が読んで分かるように加筆・補足して掲載しています
テキストベースの思考整理ツール「アイディア・レーン」最新版はこちら。
コメント