2023/05/08
- Firebase関連のファイルを組み込み。実装はまだ。
2023/05/09
- レーンの高さ最適化機能実装のトライアル。
- ウィンドウサイズを変えたときに、レーンの高さも自動で変わるようにするようにした。
2023/05/11
レーンを超えたアイディアの移動を実装。横レーン追加によりこれまでの挙動を結構見直すことになった。
キーボード操作としてはCtrl+矢印を割り当てていたが、いったんAlt+カーソルキーに変更した。かつShift+Alt+カーソルキーで明示的にレーンを超えた移動をすべく挙動を修正中。
2023/05/12
レーンを超えたアイディアの移動の続き。だいぶマシになってきた。
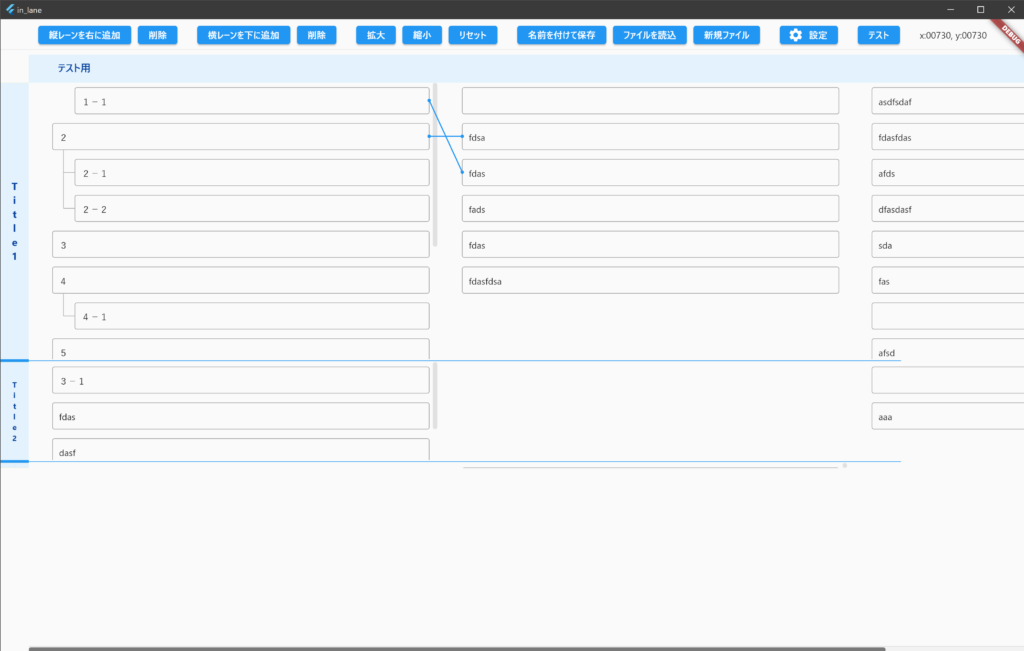
2023/05/13
レーンを超えたアイディアの移動の続き。フォーカスの移動がうまく反映されないなどかなり苦労したが、おおむね形になった。
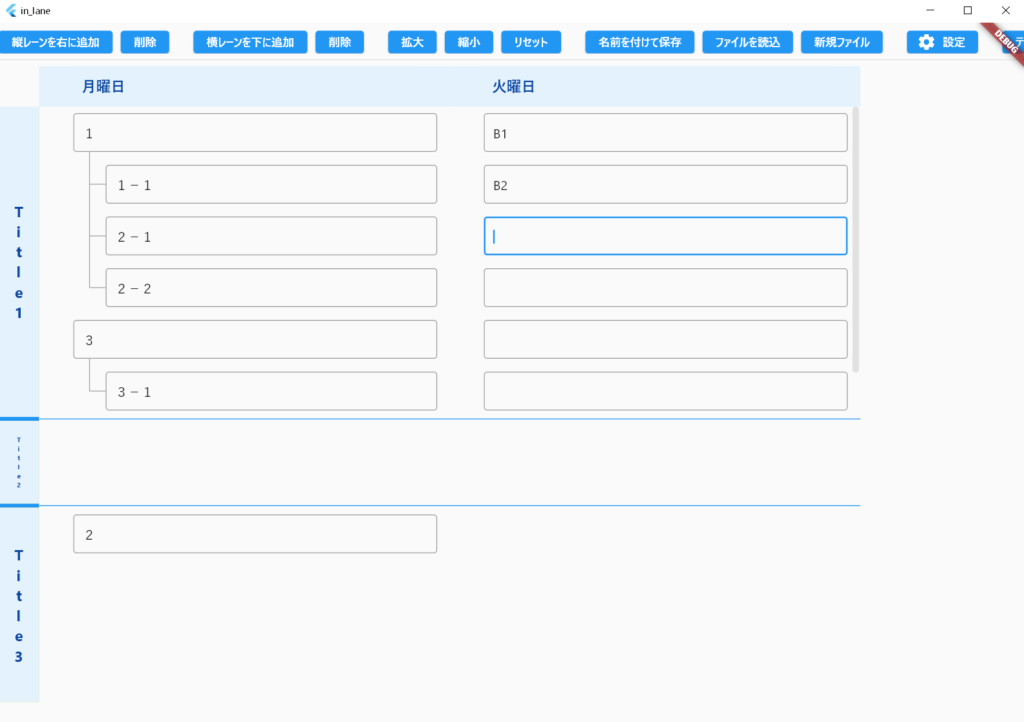
この時点でのflutterソースファイル:main.dart
import 'package:flutter/material.dart';
import 'package:flutter/services.dart';
import 'package:flutter_riverpod/flutter_riverpod.dart';
import 'logic_collection.dart';
import 'settings.dart' as setting_dart;
import 'dart:math';
import 'dart:ui';
import 'dart:convert'; //JSON形式のデータを扱う
import 'package:path/path.dart' as path_dart; //asが無いとcontextを上書きされてしまう
import 'package:file_selector/file_selector.dart';
class MyCustomScrollBehavior extends MaterialScrollBehavior {
@override
Set<PointerDeviceKind> get dragDevices => {
PointerDeviceKind.touch, // 通常のタッチ入力デバイス
PointerDeviceKind.mouse, // これを追加! https://qiita.com/Kurunp/items/5112b72e618002a93939
};
}
void main() => runApp(ProviderScope(child: MyApp()));
class MyApp extends StatelessWidget {
final GlobalKey<_WholeAppState> wholeAppKey = GlobalKey();
MyApp({super.key});
@override
Widget build(BuildContext context) {
return MaterialApp(
//scrollBehavior: MyCustomScrollBehavior(),
home: NotificationListener<SizeChangedLayoutNotification>(
onNotification: (notification) {
print("window size changed");
wholeAppKey.currentState!.canvasZoneKey.currentState!.reflectLastLaneHeight();
return true;
},
child: SizeChangedLayoutNotifier(
child: Scaffold(
body: SafeArea(child:
WholeApp(key: wholeAppKey),
),
),
),
),
);
}
}
class WholeApp extends StatefulWidget {
const WholeApp({Key? key}) : super(key: key);
@override
State<WholeApp> createState() => _WholeAppState();
}
class _WholeAppState extends State<WholeApp> {
GlobalKey<CanvasZoneState> canvasZoneKey = GlobalKey();
GlobalKey<ToolbarZoneState> toolbarZoneKey = GlobalKey();
late ToolbarZone toolbarZone;
late CanvasZone canvasZone;
double x = 0.0;
double y = 0.0;
@override
void initState() {
canvasZone = CanvasZone(key: canvasZoneKey);
toolbarZone = ToolbarZone(key: toolbarZoneKey);
super.initState();
}
void _updateMouseLocation(PointerEvent details) {
setState(() {
toolbarZoneKey.currentState!.mouseX = details.position.dx;
toolbarZoneKey.currentState!.mouseY = details.position.dy;
});
}
@override
Widget build(BuildContext context) {
return WholeAppInheritedWidget(
wholeAppState: this,
child: MouseRegion(
onHover: _updateMouseLocation, //座標を表示する
child: Column(
children: [
toolbarZone,
const Divider(),
canvasZone,
]
),
),
);
}
}
class WholeAppInheritedWidget extends InheritedWidget {
final _WholeAppState wholeAppState;
const WholeAppInheritedWidget({required this.wholeAppState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(WholeAppInheritedWidget oldWidget) => true;
static _WholeAppState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<WholeAppInheritedWidget>()!.wholeAppState;
}
}
class ToolbarZone extends ConsumerStatefulWidget {
@override
final GlobalKey<ToolbarZoneState> key;
const ToolbarZone({required this.key}) : super(key: key);
@override
ToolbarZoneState createState() => ToolbarZoneState();
}
class ToolbarZoneState extends ConsumerState<ToolbarZone> {
double mouseX = -1;
double mouseY = -1;
@override
void initState() {
super.initState();
}
void addNewVerticalLane() {
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.addNewVerticalLane();
}
void removeVerticalLane() {
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.setState(() {
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.removeLastVerticalLane();
});
}
void removeHorizontalLane() => WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.removeLastHorizontalLane();
void addNewHorizontalLane() => WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.addNewHorizontalLane();
void test() => WholeAppInheritedWidget.of(context).canvasZoneKey.currentState?.test();
void callSettingsScreen() async {
await setting_dart.SettingDialog(context);
setState(() {});
}
void scaleUp() {
ref.watch(currentScale.notifier).state = ref.read(currentScale)*1.2;
}
void scaleDown() {
ref.watch(currentScale.notifier).state = ref.read(currentScale)*0.85;
}
void scaleReset() {
if (WholeAppInheritedWidget.of(context).canvasZoneKey.currentState == null) return;
TransformationController controller = WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!._transformationController;
controller.value = Matrix4.identity();
ref.watch(currentScale.notifier).state = 1.0;
}
void newCanvas() {
if (WholeAppInheritedWidget.of(context).canvasZoneKey.currentState == null) return;
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.clearCurrentData();
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.addNewHorizontalLane();
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.addNewVerticalLane();
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.updateCanvasParametersAndRequestFocusForInit();
}
Future<void> loadData({String filename =''}) async {
await loadDataFromFile(fileName: filename, state: WholeAppInheritedWidget.of(context).canvasZoneKey.currentState);
//レーンの高さを取得
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.updateAllHorizontalViewTopAndLaneHeights();
//スクロールポジションを戻す
//WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!._scrollController.jumpTo(0);
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.setState(() {}); //画面の再描画
}
Future<void> loadDataWithDialog() async {
const XTypeGroup typeGroup = XTypeGroup(
label: 'json',
extensions: ['json'],
);
final XFile? file = await openFile(acceptedTypeGroups: [typeGroup]);
if (file != null) {
await loadData(filename:file.path);
//レーンの数に合わせて全体の高さや見た目を調整
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.optimizeHorizontalViewAndHeights();
//現在のレーンの高さを計算&反映
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.updateAllHorizontalViewTopAndLaneHeights();
//画面の描画とフォーカスの設定
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.setState(() {}); //画面の再描画
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.firstVerticalLanes[0].ideas[0].key.currentState?._textFocusNode.requestFocus();
}
}
Future<void> saveAs() async {
String? path = await getSavePath(
acceptedTypeGroups: [
const XTypeGroup(label: 'json', extensions: ['json'])
],
suggestedName: "",
);
if (path != null) {
if (path_dart.extension(path) == '') {
path = '$path.json';
}
saveDataToFile(fileName: path, state: WholeAppInheritedWidget.of(context).canvasZoneKey.currentState);
}
}
String getCurrentMousePositionStr() {
String result = "";
if (mouseX != -1) {
result = "x:${mouseX.toInt().toString().padLeft(5,'0')}, y:${mouseY.toInt().toString().padLeft(5,'0')} ";
}
return result;
}
@override
Widget build(BuildContext context) {
return ToolbarZoneInheritedWidget(
toolbarZoneState: this,
child: Column(children: [
Align(
alignment: Alignment.center,
child: SingleChildScrollView(
scrollDirection: Axis.horizontal,
child: Container( //ツールバー
margin: const EdgeInsets.only(top: 10),
child: Row(
mainAxisAlignment: MainAxisAlignment.center,
children: [
ElevatedButton(
onPressed: addNewVerticalLane,
child: const Text("縦レーンを右に追加"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: removeVerticalLane,
child: const Text("削除"),
),
const SizedBox(width: 30),
ElevatedButton(
onPressed: addNewHorizontalLane,
child: const Text("横レーンを下に追加"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: removeHorizontalLane,
child: const Text("削除"),
),
const SizedBox(width: 30),
ElevatedButton(
onPressed: scaleUp,
child: const Text("拡大"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: scaleDown,
child: const Text("縮小"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: scaleReset,
child: const Text("リセット"),
),
const SizedBox(width: 30),
ElevatedButton(
onPressed: saveAs,
child: const Text("名前を付けて保存"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: loadDataWithDialog,
child: const Text("ファイルを読込"),
),
const SizedBox(width: 10),
ElevatedButton(
onPressed: newCanvas,
child: const Text("新規ファイル"),
),
const SizedBox(width: 30),
ElevatedButton.icon(
onPressed: callSettingsScreen,
icon: const Icon(Icons.settings),
label: const Text("設定"),
),
const SizedBox(width: 30),
ElevatedButton(
onPressed: test,
child: const Text("テスト"),
),
const SizedBox(width: 30),
Text(getCurrentMousePositionStr()),
],
),
),
),
),
]),
);
}
}
class ToolbarZoneInheritedWidget extends InheritedWidget {
final ToolbarZoneState toolbarZoneState;
const ToolbarZoneInheritedWidget({required this.toolbarZoneState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(ToolbarZoneInheritedWidget oldWidget) => true;
static ToolbarZoneState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<ToolbarZoneInheritedWidget>()!.toolbarZoneState;
}
}
class HorizontalLane {
int index = 0;
double viewHeight = GlobalConst.horizontalLaneDefaultViewHeight;
double realHeight = GlobalConst.horizontalLaneDefaultViewHeight;
double viewTop = 0;
late HorizontalLaneHeader header;
double getRealHeight() {
double result = 1;
for (var element in childVLaneBodies) {
if( element.requiredHeight > result ) {
result = element.requiredHeight;
}
}
return result;
}
List<LaneBody> childVLaneBodies = [];
HorizontalLane(this.index, String title) {
header = HorizontalLaneHeader(index: index, relatedLane: this, title: title,);
}
}
class CanvasZone extends ConsumerStatefulWidget {
const CanvasZone({Key? key}) : super(key: key);
@override
CanvasZoneState createState() => CanvasZoneState();
}
class CanvasZoneState extends ConsumerState<CanvasZone> {
List<HorizontalLane> horizontalLanes = [];
double horizontalLaneHeaderWidth = GlobalConst.horizontalLaneHeaderDefaultWidth;
double allSumHorizontalViewHeights=500;
List<LaneBody> firstVerticalLanes = [];
List<VerticalLaneHeader> verticalLaneHeaders = [];
final TransformationController _transformationController = TransformationController();
final GlobalKey _box1Key = GlobalKey();
final GlobalKey _box2Key = GlobalKey();
double globalTop = 0.0;
final _scrollControllerVertical = ScrollController();
final _scrollControllerHorizontal = ScrollController();
double dx=0;
double laneWidth = 100;
@override
void initState() {
super.initState();
WidgetsBinding.instance.addPostFrameCallback((_) {
() async{
addNewHorizontalLane();
addNewVerticalLane();
await loadDataAtStartUp();
updateCanvasParametersAndRequestFocusForInit();
}();
});
}
Future<void> loadDataAtStartUp() async {
await loadDataFromFile(state: this);
}
void test() {
setState(() {});
print("MediaQuery.of(context).size.height:${MediaQuery.of(context).size.height}");
print("globalTop:${globalTop}");
print("ref.watch(verticalHeaderHeight):${ref.watch(verticalHeaderHeight)}");
print("ref.watch(currentScale):${ref.watch(currentScale)}");
print("horizontalLanes.length:${horizontalLanes.length}");
for(int i=0; i< horizontalLanes.length; i++) {
print("---");
print("horizontalLanes[$i].index title:${horizontalLanes[i].index} ${horizontalLanes[i].header.title}");
print("horizontalLanes[$i].viewTop:${horizontalLanes[i].viewTop}");
print("horizontalLanes[$i].viewHeight:${horizontalLanes[i].viewHeight}");
print("horizontalLanes[$i].childVLaneBodies.length:${horizontalLanes[i].childVLaneBodies.length}");
for(int v=0; v< horizontalLanes[i].childVLaneBodies.length; v++) {
print("horizontalLanes[$i].childVLaneBodies[$v].indexY indexX:${horizontalLanes[i].childVLaneBodies[v].indexY} ${horizontalLanes[i].childVLaneBodies[v].indexX}");
print("horizontalLanes[$i].childV"
""
"LaneBodies[$v].ideas.length:${horizontalLanes[i].childVLaneBodies[v].ideas.length}");
}
}
}
void clearCurrentData() {
for(int h=horizontalLanes.length-1; h>=0; h--) {
removeLastHorizontalLane();
}
verticalLaneHeaders.clear();
horizontalLanes.clear();
horizontalLaneHeaderWidth = GlobalConst.horizontalLaneHeaderDefaultWidth;
ref.watch(ideasRelationList.notifier).state.clear();
}
void updateCanvasParametersAndRequestFocusForInit() {
getMaxLanesHeight();
//CanvasのTop座標を取得(スクロール制御用)
globalTop = (WholeAppInheritedWidget.of(context).canvasZoneKey.currentContext!.findRenderObject() as RenderBox).localToGlobal(Offset.zero).dy;
//レーンの幅を決定
ref.watch(eachLaneWidth.notifier).state = MediaQuery.of(context).size.width * 0.4;
laneWidth = MediaQuery.of(context).size.width * 0.4;
//レーンの数に合わせて全体の高さや見た目を調整
optimizeHorizontalViewAndHeights();
//現在のレーンの高さを計算&反映
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.updateAllHorizontalViewTopAndLaneHeights();
//画面の描画とフォーカスの設定
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.setState(() {}); //画面の再描画
firstVerticalLanes[0].ideas[0].key.currentState?._textFocusNode.requestFocus();
}
double getMaxLanesHeight() {
int maxIdeaLength = 1;
for (var lane in firstVerticalLanes) {
if (lane.ideas.length > maxIdeaLength ) {
maxIdeaLength = lane.ideas.length;
}
}
return maxIdeaLength * GlobalConst.eachIdeaHeight;
}
String getDefaultVertLaneTitle(int index) {
return '縦レーン$index';
}
double getAllCanvasViewHeightMinusBottomSpace() {
return (MediaQuery.of(context).size.height - globalTop - (ref.watch(verticalHeaderHeight)*ref.watch(currentScale)) - 35/*余白*/) * (1 / ref.watch(currentScale));
}
void reflectLastLaneHeight() {
optimizeHorizontalViewAndHeights();
updateAllHorizontalViewTopAndLaneHeights();
}
//レーンの数に合わせて全体の高さや見た目を調整
void optimizeHorizontalViewAndHeights() {
const minimumRealHeight = GlobalConst.eachIdeaHeight *1.6;
double allCanvasViewHeightMinusBottomSpace = getAllCanvasViewHeightMinusBottomSpace();
//横レーンの高さの調整
if(horizontalLanes.length == 1) {
//レーンが1つの場合
horizontalLanes[0].viewHeight = allCanvasViewHeightMinusBottomSpace;
} else {
//複数レーンがある状態
var restHeight = allCanvasViewHeightMinusBottomSpace;
if (horizontalLanes[0].getRealHeight() <= allCanvasViewHeightMinusBottomSpace * 0.6) {
horizontalLanes[0].viewHeight = max(minimumRealHeight, horizontalLanes[0].getRealHeight());
} else {
horizontalLanes[0].viewHeight = allCanvasViewHeightMinusBottomSpace * 0.6;
}
restHeight = restHeight - horizontalLanes[0].viewHeight;
for (int i=1; i<horizontalLanes.length; i++){
if(restHeight <= 0) break;
if(i==horizontalLanes.length-1) {
//最後のレーンの場合
horizontalLanes[i].viewHeight = restHeight;
} else {
horizontalLanes[i].viewHeight = min( max(minimumRealHeight, horizontalLanes[i].getRealHeight()), restHeight);
}
restHeight = restHeight - horizontalLanes[i].viewHeight;
}
}
//横レーンのヘッダー領域の幅
if( (horizontalLanes.length == 1) &&
(
(horizontalLanes[0].header.title == '') ||
(horizontalLanes[0].header.title == getDefaultVertLaneTitle(0))
) ){
horizontalLaneHeaderWidth = GlobalConst.horizontalLaneHeaderDefaultWidth / 2;
} else {
horizontalLaneHeaderWidth = GlobalConst.horizontalLaneHeaderDefaultWidth;
}
}
List<Widget> getHorizontalLanesHeaders() {
List<Widget> result = [];
for(int i=0; i<horizontalLanes.length; i++) {
if(i != 0) {
result.add(
Positioned( //区切り線
left:0,
top: horizontalLanes[i-1].viewTop + horizontalLanes[i-1].viewHeight,
child: MouseRegion(
cursor: SystemMouseCursors.resizeRow,
child: GestureDetector(
onPanUpdate: (details) {
setState(() {
horizontalLanes[i-1].viewHeight = horizontalLanes[i-1].viewHeight + details.delta.dy;
updateAllHorizontalViewTop();
});
},
child: Container(
width: horizontalLaneHeaderWidth,
height: GlobalConst.horizontalLaneSplitterHeight,
color: Colors.blue,
),
),
),
)
);
}
result.add(
Positioned( //ヘッダー
left: 0,
top: horizontalLanes[i].viewTop,
child: Container(
height: horizontalLanes[i].viewHeight,
width: horizontalLaneHeaderWidth,
color: Colors.yellow,
child: horizontalLanes[i].header,
),
),
);
}
return result;
}
LaneBody addNewVLaneBodyTo(HorizontalLane targetHLane, {bool withIdea = true}){
var indexX = targetHLane.childVLaneBodies.length;
var newLane = LaneBody(indexY:targetHLane.index, indexX: indexX, ideas: []);
if(withIdea) {
newLane.ideas.add(Idea(index: 0, parentLane: newLane));
newLane.updateRequiredHeight();
}
targetHLane.childVLaneBodies.add(newLane);
return newLane;
}
Future<void> addNewVerticalLane({int horizontalLaneIndex = 0, bool withIdea = true}) async {
setState(() {
var newLane = addNewVLaneBodyTo(horizontalLanes[horizontalLaneIndex], withIdea:withIdea);
if (newLane.indexX >= verticalLaneHeaders.length) { //ヘッダーが不足している場合
verticalLaneHeaders.add( VerticalLaneHeader(index: newLane.indexX, relatedLane: newLane) );
}
});
}
updateAllHorizontalViewTop() {
//Topの計算
double aViewTop=0;
for (int i=0; i< horizontalLanes.length; i++) {
if (i==0) {
aViewTop = 0;
} else {
aViewTop = aViewTop + horizontalLanes[i-1].viewHeight + (GlobalConst.horizontalLaneSplitterHeight);
}
horizontalLanes[i].viewTop = aViewTop;
}
}
updateAllHorizontalViewTopAndLaneHeights() {
updateAllHorizontalViewTop();
//レーン全体の高さの計算
double allHeights = 0;
for (int i=0; i< horizontalLanes.length; i++) {
if( i!=0 ) {
allHeights = allHeights + GlobalConst.horizontalLaneSplitterHeight;
}
allHeights = allHeights + horizontalLanes[i].viewHeight;
}
allSumHorizontalViewHeights = allHeights;
ref.watch(lanesBodyCanvasHeight.notifier).state = WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.getMaxLanesHeight();
}
void addNewHorizontalLane({bool withIdea = true}) {
setState(() {
horizontalLanes.add( HorizontalLane( horizontalLanes.length, 'Title'+(horizontalLanes.length+1).toString()) );
addNewVLaneBodyTo(horizontalLanes[horizontalLanes.length-1],withIdea:withIdea);
});
optimizeHorizontalViewAndHeights();
updateAllHorizontalViewTopAndLaneHeights();
}
Future<void> addNewLaneAndFocusTitle(int targetLaneIndex) async {
if (targetLaneIndex == firstVerticalLanes.length) {
await addNewVerticalLane();
}
// ウィジェットが構築されるのを待つ
WidgetsBinding.instance.addPostFrameCallback((_) {
verticalLaneHeaders[targetLaneIndex].key.currentState?._titleFocusNode.requestFocus();
});
}
void removeLastVerticalLane() {
if (verticalLaneHeaders.length > 1) {
for(int h=0; h<horizontalLanes.length; h++){
//各横レーンで、現在一番右のレーンに該当するものがあれば削除する
if(horizontalLanes[h].childVLaneBodies.length == verticalLaneHeaders.length) {
var targetVLaneBody = horizontalLanes[h].childVLaneBodies[ horizontalLanes[h].childVLaneBodies.length-1 ];
//関係するリレーションを削除
for (var idea in targetVLaneBody.ideas) {
targetVLaneBody.key.currentState?.removeFromRelation(idea);
}
horizontalLanes[h].childVLaneBodies.removeLast();
}
}
verticalLaneHeaders.removeLast();
}
}
void removeLastHorizontalLane() {
setState(() {
if (horizontalLanes.length > 1) {
var targetHorizontalLane = horizontalLanes[horizontalLanes.length-1];
for(int i=targetHorizontalLane.childVLaneBodies.length-1 ; i>=0 ; i--) {
//関係するリレーションを削除
for (var idea in targetHorizontalLane.childVLaneBodies[i].ideas) {
targetHorizontalLane.childVLaneBodies[i].key.currentState?.removeFromRelation(idea);
}
}
targetHorizontalLane.childVLaneBodies.clear();
horizontalLanes.remove(targetHorizontalLane);
optimizeHorizontalViewAndHeights();
updateAllHorizontalViewTopAndLaneHeights();
}
});
}
void jumpFocusTo(int targetHLaneIndex, int targetVLaneIndex, int targetIdeaIndex, {bool fillToIndex = true}) {
if( targetHLaneIndex < 0 || targetVLaneIndex < 0 || targetIdeaIndex < 0 ) return;
if(fillToIndex == false) {
if(horizontalLanes.length <= targetHLaneIndex) {
targetIdeaIndex=0;
} else if ( horizontalLanes[targetHLaneIndex].childVLaneBodies.length <= targetVLaneIndex ) {
targetIdeaIndex=0;
} else if ( horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex].ideas.length <= targetIdeaIndex) {
targetIdeaIndex= max(0, horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex].ideas.length -1);
}
}
if( checkAndFillIdeas(targetHLaneIndex, targetVLaneIndex, targetIdeaIndex, true) ) {
horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex].rebuildItemIndexesAndUpdateRequiredHeight(0);
WidgetsBinding.instance.addPostFrameCallback((_) {
horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex].ideas[targetIdeaIndex].key.currentState?._textFocusNode.requestFocus(); //FillIdeasされた場合は遅延でのフォーカスが必要
});
} else {
horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex].ideas[targetIdeaIndex].key.currentState?._textFocusNode.requestFocus(); //FillIdeasされなかった場合は即座にフォーカス
}
}
//対象レーンに十分なアイディアがあるかチェックして、必要なら埋める
bool checkAndFillIdeas(int targetHLaneIndex, int targetVLaneIndex, int targetIdeaIndex, bool fillToIndex) {
bool added = false; //アイディアが追加されたかどうかで、呼び出し元が処理を分けられるようにする
if (targetVLaneIndex < 0 || targetIdeaIndex < 0) return added;
while (targetHLaneIndex >= horizontalLanes.length) {
//新規に横レーン追加が必要な場合
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.addNewHorizontalLane(withIdea: false);
}
while (horizontalLanes[targetHLaneIndex].childVLaneBodies.length <= targetVLaneIndex ) {
//対象横レーンに、縦レーンが足りない場合は足す
addNewVerticalLane( horizontalLaneIndex:targetHLaneIndex ,withIdea: false);
}
var targetVLane = horizontalLanes[targetHLaneIndex].childVLaneBodies[targetVLaneIndex];
//アイディアが足りなければ足す
for (int i =targetVLane.ideas.length; i <= targetIdeaIndex - (fillToIndex ? 0:1) ; i++) {
//addNewIdeaが使えない場合があるので、やむなく自力実装
targetVLane.ideas.add(Idea(index: i, parentLane: targetVLane));
added = true;
}
setState(() {});
return added;
}
@override
Widget build(BuildContext context) {
return CanvasZoneInheritedWidget(
canvasZoneState: this,
child: Expanded( //これが無いと画面描画のOverFlowを起こす
child: Stack(
children:[
Positioned(
top: 0,
left:0,
child: Transform.scale(
alignment: Alignment.topLeft,
scale: ref.watch(currentScale),
child: Container( //Canvasの全体サイズ。但し拡大縮小した場合にはサイズを補正する
width: max( MediaQuery.of(context).size.width * (1 / ref.watch(currentScale)), ref.watch(eachLaneWidth) * verticalLaneHeaders.length ),
height: max((MediaQuery.of(context).size.height - globalTop) * (1 / ref.watch(currentScale)), ( ref.watch(verticalHeaderHeight) + allSumHorizontalViewHeights/* ref.watch(lanesBodyCanvasHeight)*/ ) * (1 / ref.watch(currentScale)) ),
color: Colors.transparent, // カラーを指定しないと上のGestureDetectorの範囲がchildの範囲に収まってしまう
child: Stack(
children: [
Positioned( // 縦レーンのタイトル行(横並び)
top:0,
left: horizontalLaneHeaderWidth,
child: Container( //タイトル全体行のビューポート
width: max( ref.watch(eachLaneWidth) * verticalLaneHeaders.length, MediaQuery.of(context).size.width * (1 / ref.watch(currentScale)) ),
height: ref.watch(verticalHeaderHeight),
child: Stack(
children: [
Positioned(
top: 0,
left: ref.watch(canvasScrollDeltaX),
width: ref.watch(eachLaneWidth) * verticalLaneHeaders.length,
height:ref.watch(verticalHeaderHeight),
child: Row( children: verticalLaneHeaders, ), //縦レーン各タイトルの描画
),
],
),
),
),
Positioned( // 横レーンのタイトル行(縦並び)
top: ref.watch(verticalHeaderHeight),
left:0,
child: Container( //タイトル行全体のビューポート
width: GlobalConst.horizontalLaneHeaderDefaultWidth,
height: allSumHorizontalViewHeights,
// color: Colors.lime,
child: Stack(
children: [
Positioned(
top: 0,
left: 0,
width: GlobalConst.horizontalLaneHeaderDefaultWidth,
height: allSumHorizontalViewHeights,
child: Stack(
children: getHorizontalLanesHeaders(),//横レーン各タイトルの描画
),
),
],
),
),
),
Positioned( // ボディ部
top: ref.watch(verticalHeaderHeight),
left:horizontalLaneHeaderWidth,
child: GestureDetector(
onPanUpdate: (details) {
_scrollControllerVertical.jumpTo(_scrollControllerVertical.position.pixels - details.delta.dy);
_scrollControllerHorizontal.jumpTo(_scrollControllerHorizontal.position.pixels - details.delta.dx);
//タイトル行を一緒に動かす
double limitX = (ref.watch(eachLaneWidth) * verticalLaneHeaders.length)* ref.watch(currentScale) - MediaQuery.of(context).size.width;
limitX = limitX * (1/ ref.watch(currentScale));// + (ref.watch(eachLaneWidth)*1/5); //スケールが拡大するごとにずれていくので調整+スクロール用バッファ
if(limitX<0) {
ref.watch(canvasScrollDeltaX.notifier).state = 0;
} else {
ref.watch(canvasScrollDeltaX.notifier).state += details.delta.dx;
ref.watch(canvasScrollDeltaX.notifier).state = ref.watch(canvasScrollDeltaX).clamp( -limitX, 0 );
}
/*
print("------");
print("details.delta.dx:${details.delta.dx.toInt()}");
print("lanesBodyCanvasHeight*currentScale:${(ref.watch(lanesBodyCanvasHeight) * ref.watch(currentScale)).toInt()} (${ref.watch(lanesBodyCanvasHeight)}) * (${ref.watch(currentScale)})");
print("MediaQuery.of(context).size.height - globalTop - ( ref.watch(headerHeight)) * ref.watch(currentScale) :${ MediaQuery.of(context).size.height - globalTop - ( ref.watch(headerHeight)) * ref.watch(currentScale) }");
print("MediaQuery.of(context).size.height:${MediaQuery.of(context).size.height} globalTop:${globalTop} headerHeight:${ref.watch(headerHeight)}");
print("ref.watch(lanesBodyCanvasHeight):${ref.watch(lanesBodyCanvasHeight)}");
print("Vertical.position:${_scrollControllerVertical.position.pixels.toInt()} Vertical.maxExtent:${_scrollControllerVertical.position.maxScrollExtent.toInt()}");
print("-");
print("Horizontal.position:${_scrollControllerHorizontal.position.pixels.toInt()} Horizontal.maxExtent:${_scrollControllerHorizontal.position.maxScrollExtent.toInt()}");
print("MediaQuery.of(context).size.width:${MediaQuery.of(context).size.width}");
print("box1.width:${_box1Key.currentContext!.size!.width.toInt()} box2.width:${_box2Key.currentContext!.size!.width.toInt()}");
print("ref.watch(eachLaneWidth):${ref.watch(eachLaneWidth)} lanes.length:${lanes.length}");
*/
},
onTap: () async {
//print("${_scrollController2.position.maxScrollExtent} ${_scrollController2.position.pixels}");
if (ref.watch(relationHoverFlg)) {
await relationSelectDialog(context,this);
setState(() {});
}
},
child: Container( //スクロール範囲のビューポート
key: _box1Key,
width: MediaQuery.of(context).size.width * (1 / ref.watch(currentScale)),
height: ( MediaQuery.of(context).size.height - globalTop - ( ref.watch(verticalHeaderHeight)*ref.watch(currentScale) ) ) * (1/ref.watch(currentScale)),
child: Scrollbar(
controller: _scrollControllerVertical,
thumbVisibility: true,
trackVisibility: false,
child: Scrollbar(
controller: _scrollControllerHorizontal,
thumbVisibility: true,
trackVisibility: true,
notificationPredicate: (notif) => notif.depth == 1, //スクロールバーを入れ子にする場合に必要なようだ
child: SingleChildScrollView(
controller: _scrollControllerVertical,
child: SingleChildScrollView(
controller: _scrollControllerHorizontal,
scrollDirection: Axis.horizontal,
child: Container( // 子に描画用のStackを配置するために土台が必要
key: _box2Key,
width: ref.watch(eachLaneWidth) * verticalLaneHeaders.length,
height: max(
(
MediaQuery.of(context).size.height - globalTop - ( (ref.watch(verticalHeaderHeight)*ref.watch(currentScale)) ) ) * (1/ref.watch(currentScale)), //レーン本体のサイズが小さいが、複数レーンのヘッダーが縦に並んでいたときの対応
allSumHorizontalViewHeights /*ref.watch(lanesBodyCanvasHeight)*/ + ( GlobalConst.eachIdeaHeight * 2/3 ) /*バッファ*/
),
color: Colors.transparent,
child:Stack(
children:
buildIdeasAndRelations(this),
),
),
),
),
),
),
),
),
),
]
),
),
),
),
]
),
),
);
}
}
class CanvasZoneInheritedWidget extends InheritedWidget {
final CanvasZoneState canvasZoneState;
const CanvasZoneInheritedWidget({required this.canvasZoneState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(CanvasZoneInheritedWidget oldWidget) => true;
static CanvasZoneState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<CanvasZoneInheritedWidget>()!.canvasZoneState;
}
}
class IdeasRelation {
Idea fromIdea;
Idea toIdea;
RelationShape fromShape=RelationShape.dot;
RelationShape toShape=RelationShape.dot;
String note = '';
IdeasRelation({required this.fromIdea, required this.toIdea});
}
class VerticalLaneHeader extends ConsumerStatefulWidget {
@override
final GlobalKey<VerticalLaneHeaderState> key = GlobalKey<VerticalLaneHeaderState>();
int index;
String title;
LaneBody relatedLane;
final double indentWidth = 40; //インデントの下げ幅
VerticalLaneHeader({
required this.index,
required this.relatedLane,
this.title = '',
}) : super(key: ValueKey(GlobalKey<VerticalLaneHeaderState>().toString()));
@override
ConsumerState<VerticalLaneHeader> createState() => VerticalLaneHeaderState();
}
class VerticalLaneHeaderState extends ConsumerState<VerticalLaneHeader> {
final TextEditingController titleController = TextEditingController();
final FocusNode _titleFocusNode = FocusNode();
@override
void initState() {
super.initState();
titleController.text = widget.title;
}
@override
Widget build(BuildContext context) {
return VerticalLaneHeaderInheritedWidget(
laneHeaderState: this,
child: SizedBox(
height: 50,
width: ref.watch(eachLaneWidth),
child: Focus(
onKey: (FocusNode node, RawKeyEvent event) {
if (event.runtimeType == RawKeyDownEvent) {
if (event.logicalKey == LogicalKeyboardKey.arrowDown) { //カーソルキー ↓
widget.relatedLane.ideas[0].key.currentState?._textFocusNode.requestFocus();
} else if (event.logicalKey == LogicalKeyboardKey.arrowRight && //カーソルキー →
titleController.selection.baseOffset == titleController.text.length) {
CanvasZoneInheritedWidget.of(context).addNewLaneAndFocusTitle(widget.index + 1);
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft && //カーソルキー ←
titleController.selection.baseOffset == 0) {
if (widget.index > 0) {
CanvasZoneInheritedWidget.of(context).verticalLaneHeaders[widget.index - 1].key.currentState?._titleFocusNode.requestFocus();
}
}
}
return KeyEventResult.ignored;
},
child: Container( //タイトル
color: Colors.blue[50],
padding: const EdgeInsets.symmetric(horizontal: GlobalConst.ideaIndentWidth),
child: TextField(
autofocus: true,
controller: titleController,
focusNode: _titleFocusNode,
decoration: InputDecoration(
border: InputBorder.none,
disabledBorder: const OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 2.0),
),
fillColor: Colors.blue[50],
filled: true,
),
style: TextStyle(fontSize: 18.0, fontWeight: FontWeight.bold, color: Colors.blue[900]),
keyboardType: TextInputType.text,
textInputAction: TextInputAction.done,
onSubmitted: (value) {
_titleFocusNode.unfocus();
},
),
)
),
),
);
}
}
class VerticalLaneHeaderInheritedWidget extends InheritedWidget {
final VerticalLaneHeaderState laneHeaderState;
const VerticalLaneHeaderInheritedWidget({required this.laneHeaderState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(VerticalLaneHeaderInheritedWidget oldWidget) => true;
static VerticalLaneHeaderState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<VerticalLaneHeaderInheritedWidget>()!.laneHeaderState;
}
}
class HorizontalLaneHeader extends ConsumerStatefulWidget {
@override
final GlobalKey<HorizontalLaneHeaderState> key = GlobalKey<HorizontalLaneHeaderState>();
int index;
String title;
HorizontalLane relatedLane;
HorizontalLaneHeader({
required this.index,
required this.relatedLane,
this.title = '',
}) : super(key: ValueKey(GlobalKey<HorizontalLaneHeaderState>().toString()));
@override
ConsumerState<HorizontalLaneHeader> createState() => HorizontalLaneHeaderState();
}
class HorizontalLaneHeaderState extends ConsumerState<HorizontalLaneHeader> {
final TextEditingController titleController = TextEditingController();
final FocusNode _titleFocusNode = FocusNode();
double _opacity=1.0;
@override
void initState() {
super.initState();
titleController.text = widget.title;
}
@override
Widget build(BuildContext context) {
return HorizontalLaneHeaderInheritedWidget(
laneHeaderState: this,
child: Container(
height: widget.relatedLane.viewHeight,
width: GlobalConst.horizontalLaneHeaderDefaultWidth,
color: Colors.blue[50],
child: Focus(
onKey: (FocusNode node, RawKeyEvent event) {
return KeyEventResult.ignored;
},
child: GestureDetector(
onTap: () async {
await horizontalLaneHeaderEditDialog(context,this);
setState(() {});
},
child: MouseRegion(
onEnter: (_) {
setState(() {
_opacity = 0.9;
});
},
onExit: (_) {
setState(() {
_opacity = 1.0;
});
},
child: Opacity(
opacity: _opacity,
child: FittedBox( //親コンテナ(横レーンのViewHeight)に合わせて文字を縮小させるため
fit: BoxFit.scaleDown,
child: Container( //タイトル
padding: const EdgeInsets.symmetric(vertical: GlobalConst.ideaIndentWidth),
child: Align(
alignment: Alignment.center,
child: Tategaki(
widget.title,
style: TextStyle(fontSize: 18.0, fontWeight: FontWeight.bold, color: Colors.blue[900]),
),
),
/*
child: TextField(
autofocus: true,
controller: titleController,
focusNode: _titleFocusNode,
decoration: InputDecoration(
border: InputBorder.none,
disabledBorder: const OutlineInputBorder(
borderSide: BorderSide(color: Colors.blue, width: 2.0),
),
fillColor: Colors.blue[50],
filled: true,
),
style: TextStyle(fontSize: 18.0, fontWeight: FontWeight.bold, color: Colors.blue[900]),
keyboardType: TextInputType.text,
textInputAction: TextInputAction.done,
onSubmitted: (value) {
_titleFocusNode.unfocus();
},
),
*/
),
),
),
),
)
),
),
);
}
}
class HorizontalLaneHeaderInheritedWidget extends InheritedWidget {
final HorizontalLaneHeaderState laneHeaderState;
const HorizontalLaneHeaderInheritedWidget({required this.laneHeaderState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(HorizontalLaneHeaderInheritedWidget oldWidget) => true;
static HorizontalLaneHeaderState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<HorizontalLaneHeaderInheritedWidget>()!.laneHeaderState;
}
}
class LaneBody extends ConsumerStatefulWidget {
@override
final GlobalKey<LaneBodyState> key = GlobalKey<LaneBodyState>();
int indexX;
int indexY;
String title;
List<Idea> ideas;
double requiredHeight=0;
ScrollController scrollController = ScrollController();
LaneBody({
required this.indexX,
required this.indexY,
required this.ideas,
this.title = '',
}) : super(key: ValueKey(GlobalKey<LaneBodyState>().toString()));
void updateRequiredHeight() {
requiredHeight = ideas.length * GlobalConst.eachIdeaHeight;
}
void rebuildItemIndexesAndUpdateRequiredHeight(int startIndex) {
for (int i = startIndex; i < ideas.length; i++) {
ideas[i].index = i;
}
updateRequiredHeight();
}
@override
ConsumerState<LaneBody> createState() => LaneBodyState();
}
class LaneBodyState extends ConsumerState<LaneBody> {
List<Idea> get ideas => widget.ideas;
final TextEditingController titleController = TextEditingController();
void addNewIdea(int currentIndex) {
setState(() {
int currentIndentLevel = ideas[currentIndex].indentLevel;
ideas.insert(
currentIndex + 1,
Idea(
index: currentIndex + 1,
parentLane: widget,
indentLevel: currentIndentLevel,
),
);
rebuildItemIndexes(currentIndex + 2);
});
}
void addNewIdeaWithFocus(int currentIndex) {
addNewIdea(currentIndex);
CanvasZoneInheritedWidget.of(context).setState(() {}); //画面の再描画
WidgetsBinding.instance.addPostFrameCallback((_) {
ideas[currentIndex + 1].key.currentState?._textFocusNode.requestFocus();
});
}
void rebuildItemIndexes(int startIndex) {
widget.rebuildItemIndexesAndUpdateRequiredHeight(startIndex);
ref.watch(lanesBodyCanvasHeight.notifier).state = CanvasZoneInheritedWidget.of(context).getMaxLanesHeight();
}
void moveIdea(IdeaState state, int currentIdeaIndex, int direction) {
if (currentIdeaIndex + direction < 0) { //レーン内の一番上からさらに上に移動
moveAndInsertIdeaToAnotherLane(state, widget.indexY - 1, currentIdeaIndex, 0);
} else if (currentIdeaIndex + direction >= ideas.length) { //レーン内の一番上からさらに下に移動
moveAndInsertIdeaToAnotherLane(state, widget.indexY + 1, currentIdeaIndex, 0);
} else if (currentIdeaIndex + direction >= 0 && currentIdeaIndex + direction < ideas.length) {
setState(() {
Idea temp = ideas[currentIdeaIndex];
ideas[currentIdeaIndex] = ideas[currentIdeaIndex + direction];
ideas[currentIdeaIndex + direction] = temp;
ideas[currentIdeaIndex].key.currentState?.updateIndex(currentIdeaIndex);
ideas[currentIdeaIndex + direction].key.currentState?.updateIndex(currentIdeaIndex + direction);
});
}
}
void moveAndInsertIdeaToAnotherLane(IdeaState state, int targetHLaneIndex, int currentIdeaIndex, int vLaneDirection) {
if(targetHLaneIndex < 0 || widget.indexX + vLaneDirection < 0) return;
state._textFocusNode.unfocus(); //後でフォーカスを正しく反映させるために必要
List<HorizontalLane> horizontalLanes = CanvasZoneInheritedWidget.of(context).horizontalLanes;
LaneBody targetLaneBody;
int targetIdeaIndex;
if(vLaneDirection == 0) { //上下に移動の場合
CanvasZoneInheritedWidget.of(context).checkAndFillIdeas( targetHLaneIndex, widget.indexX + vLaneDirection, 0, false);
targetLaneBody = horizontalLanes[targetHLaneIndex].childVLaneBodies[widget.indexX];
targetIdeaIndex = targetLaneBody.ideas.length;
} else { //左右に移動の場合
CanvasZoneInheritedWidget.of(context).checkAndFillIdeas( targetHLaneIndex, widget.indexX + vLaneDirection, currentIdeaIndex, false);
targetLaneBody = horizontalLanes[targetHLaneIndex].childVLaneBodies[widget.indexX + vLaneDirection];
targetIdeaIndex = currentIdeaIndex;
}
//新レーンへアイディアを移動
targetLaneBody.ideas.insert(targetIdeaIndex, ideas[currentIdeaIndex]);
targetLaneBody.ideas[targetIdeaIndex].parentLane = targetLaneBody;
targetLaneBody.rebuildItemIndexesAndUpdateRequiredHeight(0);
if (targetLaneBody.key.currentState != null) {
targetLaneBody.key.currentState!.setState(() {}); //描画更新のために必要
}
//元のレーンから削除
widget.ideas.removeAt(currentIdeaIndex);
widget.rebuildItemIndexesAndUpdateRequiredHeight(0);
if(widget.indexY == horizontalLanes.length - 1 && widget.ideas.isEmpty) {
//横レーン内の中身が全て無い場合は削除
bool ideaExistFlg = false;
for(int i=0; i<horizontalLanes[widget.indexY].childVLaneBodies.length; i++){
if(horizontalLanes[widget.indexY].childVLaneBodies[i].ideas.isNotEmpty) {
ideaExistFlg = true;
break;
}
}
if(ideaExistFlg == false) {
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.removeLastHorizontalLane();
}
}
WholeAppInheritedWidget.of(context).canvasZoneKey.currentState!.setState(() {});
WidgetsBinding.instance.addPostFrameCallback((_) { //遅延にしないとフォーカス移動が正しく反映されない
CanvasZoneInheritedWidget.of(state.context/*ここではstateのcontextを使わないとエラーになる*/).jumpFocusTo(targetLaneBody.indexY, targetLaneBody.indexX, targetIdeaIndex, fillToIndex: false);
});
}
void removeFromRelation(Idea targetIdea) {
//削除対象のリレーションを探す
List<IdeasRelation> removeTargetList = [];
if (ref.read(ideasRelationList).isNotEmpty) {
for (var relationSet in ref.read(ideasRelationList)) {
if (relationSet.fromIdea == targetIdea || relationSet.toIdea == targetIdea) {
removeTargetList.add(relationSet);
}
}
}
//実際に削除する
if (removeTargetList.isNotEmpty) {
for (var relationSet in removeTargetList) {
removeRelation(ref.watch(ideasRelationList.notifier).state, relationSet);
}
}
}
void removeIdea(int currentIndex) {
if (ideas.length > 1) {
removeFromRelation(ideas[currentIndex]);
ideas.removeAt(currentIndex);
for (int i = currentIndex; i < ideas.length; i++) {
ideas[i].index = i;
}
widget.updateRequiredHeight();
ideas[max(0, currentIndex - 1)].key.currentState?._textFocusNode.requestFocus();
CanvasZoneInheritedWidget.of(context).setState(() {}); //画面の再描画
}
}
void focusNextIdea(int currentIndex, int direction) {
if (currentIndex == 0 && direction <= 0) { //レーンを超えて上に移動
if(widget.indexY == 0) {
//タイトルにフォーカスを移す
CanvasZoneInheritedWidget.of(context).verticalLaneHeaders[widget.indexX].key.currentState!._titleFocusNode.requestFocus();
} else {
//上のレーンにアイディアがあればそこにフォーカスを移す
for(int h=widget.indexY-1; h>=0; h--) {
var targetHLane = CanvasZoneInheritedWidget.of(context).horizontalLanes[h];
if(targetHLane.childVLaneBodies.length > widget.indexX && targetHLane.childVLaneBodies[widget.indexX].ideas.isNotEmpty) {
targetHLane.childVLaneBodies[widget.indexX].ideas[
targetHLane.childVLaneBodies[widget.indexX].ideas.length -1
].key.currentState?._textFocusNode.requestFocus();
break;
}
}
}
} else if (currentIndex + direction >= ideas.length) { //レーンを超えて下に移動
//下のレーンにアイディアがあればそこにフォーカスを移す
for(int h=widget.indexY+1; h<CanvasZoneInheritedWidget.of(context).horizontalLanes.length; h++) {
var targetHLane = CanvasZoneInheritedWidget.of(context).horizontalLanes[h];
if(targetHLane.childVLaneBodies.length > widget.indexX && targetHLane.childVLaneBodies[widget.indexX].ideas.isNotEmpty) {
targetHLane.childVLaneBodies[widget.indexX].ideas[
0
].key.currentState?._textFocusNode.requestFocus();
}
}
} else if (currentIndex + direction >= 0 && currentIndex + direction < ideas.length) { //レーン内の移動
ideas[currentIndex + direction].key.currentState?._textFocusNode.requestFocus();
}
}
@override
void initState() {
super.initState();
titleController.text = widget.title;
}
@override
Widget build(BuildContext context) {
return LaneBodyInheritedWidget(
laneState: this,
child: SizedBox(
width: double.infinity,
height: (GlobalConst.eachIdeaHeight * ideas.length).toDouble(),
child: Column(
children: ideas
),
),
);
}
}
class LaneBodyInheritedWidget extends InheritedWidget {
final LaneBodyState laneState;
const LaneBodyInheritedWidget({required this.laneState, required Widget child, super.key}) : super(child: child);
@override
bool updateShouldNotify(LaneBodyInheritedWidget oldWidget) => true;
static LaneBodyState of(BuildContext context) {
return context.dependOnInheritedWidgetOfExactType<LaneBodyInheritedWidget>()!.laneState;
}
}
class Idea extends ConsumerStatefulWidget {
@override
final GlobalKey<IdeaState> key = GlobalKey<IdeaState>();
int index;
int indentLevel;
final double textFieldHeight; //テキスト欄の高さ
String content;
LaneBody parentLane;
List<Idea> relatedIdeasTo = [];
List<Idea> relatedIdeasFrom = [];
Idea({
required this.index,
required this.parentLane,
this.textFieldHeight = 48.0, //デフォルトは48
this.indentLevel = 0,
this.content = '',
}) : super(key: ValueKey(GlobalKey<IdeaState>().toString()));
@override
IdeaState createState() => IdeaState();
}
class IdeaState extends ConsumerState<Idea> with AutomaticKeepAliveClientMixin<Idea> {
final TextEditingController textController = TextEditingController();
final FocusNode _textFocusNode = FocusNode();
void updateIndex(int newIndex) {
setState(() {
widget.index = newIndex;
});
}
// インデントの線を描画する
void _indentLinePaint(Canvas canvas, Size size) {
indentLinePaint(canvas, size, context, widget.index, widget.indentLevel, widget.textFieldHeight, GlobalConst.ideaIndentWidth);
}
String toJson() {
Map<String, dynamic> data = {
'index': widget.index,
'indentLevel': widget.indentLevel,
'text': textController.text,
};
return json.encode(data);
}
void startRelationMode() {
ref.watch(relatedIdeaSelectMode.notifier).state = true;
ref.watch(originIdeaForSelectMode.notifier).state = widget;
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar( content: Text( '接続先を選んでEnterキーを押してください'),)
);
}
void endRelationMode() {
StateController<bool> mode = ref.watch(relatedIdeaSelectMode.notifier);
Idea? origin = ref.watch(originIdeaForSelectMode.notifier).state;
ScaffoldMessenger.of(context).hideCurrentSnackBar();
if (origin != null) {
bool cancelFlg = false;
List<IdeasRelation> ideasRelations = ref.watch(ideasRelationList.notifier).state;
//自分自身に接続しようとしていないかチェック
if(origin == widget) {
cancelFlg = true;
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar( content: Text('自分自身には接続できません'), ),
);
} else {
//既に同じリレーションが無いかチェック
for (var element in ideasRelations) {
if ( (element.fromIdea == origin && element.toIdea == widget) || (element.fromIdea == widget && element.toIdea == origin) ){
cancelFlg = true;
ScaffoldMessenger.of(context).showSnackBar(
const SnackBar( content: Text('既に接続されています'), ),
);
break;
}
}
}
if(cancelFlg == false) {
widget.relatedIdeasFrom.add(origin);
origin.relatedIdeasTo.add(widget);
ref.watch(ideasRelationList.notifier).state.add(IdeasRelation(fromIdea: origin, toIdea: widget));
CanvasZoneInheritedWidget.of(context).setState(() {}); //Relationの線を描画
}
origin = null;
}
mode.state = false;
}
late final Map<Type, Action<Intent>> _actionMap;
@override
void initState() {
super.initState();
textController.text = widget.content;
_actionMap = <Type, Action<Intent>>{
ActivateIntent: CallbackAction<Intent>(
onInvoke: (Intent intent) => startRelationMode(),
),
};
}
@override
bool get wantKeepAlive => true; //これが無いと画面外から出たときに中身も消えてしまう
final Map<ShortcutActivator, Intent> _shortcutMap =
const <ShortcutActivator, Intent>{
SingleActivator(LogicalKeyboardKey.keyX, control: true): ActivateIntent(),
};
//TextFieldでEnterを押した場合のキーの処理について
// ・webだと、submit→key webの順で処理される
// ・windowsだと、key → submitの順で処理される
@override
Widget build(BuildContext context) {
super.build(context);
return Focus(
onKey: (FocusNode node, RawKeyEvent event) {
//print("event.runtimeType:${event.runtimeType.toString()} Normal${event.logicalKey.keyLabel} , Web${ (event.data is RawKeyEventDataWeb) ? (event.data as RawKeyEventDataWeb).code : ''}");
if (event.runtimeType == RawKeyDownEvent) {
if ((event.logicalKey.keyLabel == 'Enter') || //Enterキー押下
( (event.data is RawKeyEventDataWeb)&&(event.data as RawKeyEventDataWeb).code == 'Enter') ) { //Enterの場合のみ特殊処理が必要
if (event.isControlPressed) {
startRelationMode();
}
else {
if (ref.read(relatedIdeaSelectMode.notifier).state == true) { //relatedの指定モード
endRelationMode();
_textFocusNode.requestFocus();
} else {
_textFocusNode.unfocus();
LaneBodyInheritedWidget.of(context).addNewIdeaWithFocus(widget.index);
}
}
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.capsLock) {
// CapsLockキーを無視する条件を追加
return KeyEventResult.ignored;
} else if (event.isControlPressed && event.isAltPressed){ // Ctrl + Alt :冗長な判定だが、設定でCtrlとAltを入れ替える処理に備え別扱いとしている
if (event.logicalKey == LogicalKeyboardKey.arrowRight) { // Ctrl + Alt + →
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY, widget.index, 1);
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft) { // Ctrl + Alt + ←
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY, widget.index, -1);
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowUp) { // Ctrl + Alt + ↑
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY - 1, widget.index, 0);
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) { // Ctrl + Alt + ↓
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY + 1, widget.index, 0);
return KeyEventResult.handled;
}
} else if (GlobalConst.swapCtrlAlt ? event.isAltPressed : event.isControlPressed) { // コントロールキーのみ押下:レーンを超えたフォーカスの移動
if (event.logicalKey == LogicalKeyboardKey.tab) { // Ctrl + TAB
startRelationMode();
} else if (event.logicalKey == LogicalKeyboardKey.arrowUp) { // Ctrl + ↑
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY -1 , widget.parentLane.indexX, widget.index, fillToIndex: false);
return KeyEventResult.handled; //余計なフォーカスの移動を防ぐ
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) { // Ctrl + ↓
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY +1 , widget.parentLane.indexX, widget.index, fillToIndex: false);
return KeyEventResult.handled; //余計なフォーカスの移動を防ぐ
} else if (event.logicalKey == LogicalKeyboardKey.arrowRight) {// Ctrl + →
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY, widget.parentLane.indexX + 1, widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft) { // Ctrl + ←
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY, widget.parentLane.indexX - 1, widget.index);
}
} else if (GlobalConst.swapCtrlAlt ? event.isControlPressed : event.isAltPressed) { // Altキー押下:アイディアの移動
if (event.logicalKey == LogicalKeyboardKey.arrowRight) { // Alt + →
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY, widget.index, 1);
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft) { // Alt + ←
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY, widget.index, -1);
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowUp) { // Alt + ↑
if (event.isShiftPressed) {
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY - 1, widget.index, 0);
} else {
LaneBodyInheritedWidget.of(context).moveIdea(this, widget.index, -1);
LaneBodyInheritedWidget.of(context).rebuildItemIndexes(0); //再度構築しなおさないと、インデックスがおかしくなる
}
return KeyEventResult.handled;
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) { // Alt + ↓
if (event.isShiftPressed) {
LaneBodyInheritedWidget.of(context).moveAndInsertIdeaToAnotherLane(this, widget.parentLane.indexY + 1, widget.index, 0);
} else {
LaneBodyInheritedWidget.of(context).moveIdea(this, widget.index, 1);
LaneBodyInheritedWidget.of(context).rebuildItemIndexes(0); //再度構築しなおさないと、インデックスがおかしくなる
}
return KeyEventResult.handled;
}
} else if (event.logicalKey == LogicalKeyboardKey.arrowUp) { //カーソルキー ↑
LaneBodyInheritedWidget.of(context).focusNextIdea(widget.index, -1);
return KeyEventResult.handled; //余計なフォーカスの移動を防ぐ
} else if (event.logicalKey == LogicalKeyboardKey.arrowDown) { //カーソルキー ↓
LaneBodyInheritedWidget.of(context).focusNextIdea(widget.index, 1);
return KeyEventResult.handled; //余計なフォーカスの移動を防ぐ
} else if (event.logicalKey == LogicalKeyboardKey.arrowRight && //カーソルキー →
textController.selection.baseOffset == textController.text.length) {
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY, widget.parentLane.indexX + 1, widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.arrowLeft && textController.selection.baseOffset == 0) { //カーソルキー ←
CanvasZoneInheritedWidget.of(context).jumpFocusTo(widget.parentLane.indexY, widget.parentLane.indexX - 1, widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.tab) { //TABキー
if (event.isShiftPressed) { //SHIFT + TABキー
setState(() {
widget.indentLevel = max(0, widget.indentLevel - 1);
});
} else {
setState(() {
widget.indentLevel += 1;
});
}
findAndRepaintAffectedIdeas(context, widget.index, widget.indentLevel, widget.index, widget.index);
return KeyEventResult.handled; //デフォルトのタブの挙動を無効化して、フォーカスの移動を防ぐ
} else if ((event.logicalKey == LogicalKeyboardKey.delete || //DELキー & BSキー
event.logicalKey == LogicalKeyboardKey.backspace) &&
textController.text.isEmpty) {
LaneBodyInheritedWidget.of(context).removeIdea(widget.index);
} else if (event.logicalKey == LogicalKeyboardKey.f1) { // F1キー
startRelationMode();
}
}
return KeyEventResult.ignored;
},
child: RawKeyboardListener(
focusNode: FocusNode(),
child: Container(
margin: const EdgeInsets.symmetric(horizontal: ideaHorizontalMargin, vertical: ideaVerticalMargin),
child: Row(
crossAxisAlignment: CrossAxisAlignment.center,
children: [
const SizedBox(width: ideaLeftSpace, child: DecoratedBox(decoration: BoxDecoration(color: Colors.red))),
Expanded(
flex: 4,
child: Row(children: [
CustomPaint(
painter: _CustomPainter(_indentLinePaint),
child: Container(width: GlobalConst.ideaIndentWidth * widget.indentLevel),
),
Expanded(
child: SizedBox(
height: widget.textFieldHeight,
child: FocusableActionDetector(
actions: _actionMap,
shortcuts: _shortcutMap,
child: TextFormField(
controller: textController,
focusNode: _textFocusNode,
maxLines: 1,
decoration: const InputDecoration(
border: OutlineInputBorder(),
),
keyboardType: TextInputType.multiline,
textInputAction: TextInputAction.done,
onFieldSubmitted: (value) {
_textFocusNode.requestFocus(); //Enterはこれが無いとKeyEventが発生しない(おそらくフォーカスが無くなるから。少なくともWebでは必須)
},
),
),
),
),
]),
),
],
),
),
)
);
}
}
class _CustomPainter extends CustomPainter {
final void Function(Canvas, Size) _paint;
_CustomPainter(this._paint);
@override
void paint(Canvas canvas, Size size) {
_paint(canvas, size);
}
@override
bool shouldRepaint(covariant CustomPainter oldDelegate) => false;
}
logic_collection.dart (main.dartと同じフォルダに配置)
import 'package:flutter/material.dart';
import 'main.dart';
import 'dart:convert'; //JSON形式のデータを扱う
import 'package:path_provider/path_provider.dart';
import 'dart:io';
import 'dart:math';
import 'package:flutter_riverpod/flutter_riverpod.dart';
//リレーションの情報
StateProvider<bool> relatedIdeaSelectMode = StateProvider((ref) => false);
StateProvider<Idea?> originIdeaForSelectMode = StateProvider((ref) => null);
StateProvider<List<IdeasRelation>> ideasRelationList = StateProvider((ref) => []);
StateProvider<IdeasRelation?> selectedRelation = StateProvider((ref) => null);
//リレーションの見た目
enum RelationShape{dot,arrow}
StateProvider<Color> relationColor = StateProvider((ref) => Colors.blue);
StateProvider<bool> relationHoverFlg = StateProvider((ref) => false);
const bool relationDebugPaint = false;
//スケール
StateProvider<double> currentScale = StateProvider((ref) => 1);
//各種ウィジェットのサイズと表現
StateProvider<double> verticalHeaderHeight = StateProvider((ref) => 50);
StateProvider<double> eachLaneWidth = StateProvider((ref) => 500);
StateProvider<double> lanesBodyCanvasHeight = StateProvider((ref) => 500);
StateProvider<double> relationDotSize = StateProvider((ref) => 6);
StateProvider<double> relationArrowSize = StateProvider((ref) => 10);
class GlobalConst {
static const eachIdeaHeight = 64.0; //48+8+8 doubleと認識させるために64.0の小数点が必要
static const horizontalLaneHeaderDefaultWidth = 50.0;
static const horizontalLaneSplitterHeight = 5.0;
static const horizontalLaneDefaultViewHeight = 350.0;
static const ideaIndentWidth = 40.0;
static const swapCtrlAlt = true;
}
const double ideaLeftSpace = 26;
const double ideaHorizontalMargin = 16;
const double ideaVerticalMargin = 8;
const Color relationColorTransparent = Colors.transparent;//デバッグ時に変えられるよう定義
//タイトル行のスクロール用
StateProvider<double> canvasScrollDeltaX = StateProvider((ref) => 0);
StateProvider<double> canvasScrollDeltaY = StateProvider((ref) => 0);
//2点間の距離を求める関数
double getDistance(double x, double y, double x2, double y2) {
double distance = sqrt((x2 - x) * (x2 - x) + (y2 - y) * (y2 - y));
return distance;
}
//2点間の角度を求める関数
double getRadian(double x, double y, double x2, double y2) {
double radian = atan2(y2 - y,x2 - x);
return radian;
}
//右向き矢印
class TrianglePainterR extends CustomPainter{
Color color;
TrianglePainterR(this.color);
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()..color = color;
// 三角(塗りつぶし)
var path = Path();
path.moveTo(0, 0);
path.lineTo(size.width, size.height / 2);
path.lineTo(0, size.height);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => false;
}
//左向き矢印
class TrianglePainterL extends CustomPainter{
Color color;
TrianglePainterL(this.color);
@override
void paint(Canvas canvas, Size size) {
var paint = Paint()..color = color;
// 三角(塗りつぶし)
var path = Path();
path.moveTo(size.width, 0);
path.lineTo(0, size.height / 2);
path.lineTo(size.width, size.height);
path.close();
canvas.drawPath(path, paint);
}
@override
bool shouldRepaint(CustomPainter oldDelegate) => false;
}
void removeRelation(List<IdeasRelation> ideasRelationList, IdeasRelation targetRelation) {
targetRelation.fromIdea.relatedIdeasTo.remove(targetRelation.toIdea);
targetRelation.toIdea.relatedIdeasFrom.remove(targetRelation.fromIdea);
ideasRelationList.remove(targetRelation);
}
Future<dynamic> horizontalLaneHeaderEditDialog(BuildContext context, HorizontalLaneHeaderState state) {
final TextEditingController textController = TextEditingController();
textController.text = state.widget.title;
return showDialog(
context: context,
builder: (context) {
return StatefulBuilder(
builder: (context, setState) {
return SimpleDialog(
contentPadding: const EdgeInsets.all(26),
children: [
Align( // 閉じるボタン
alignment: Alignment.centerRight,
child: Tooltip(
message: MaterialLocalizations
.of(context)
.closeButtonTooltip,
child: GestureDetector(
onTap: () => Navigator.pop(context),
child: const Icon(Icons.close, size: 30),
),
),
),
TextField(
controller: textController,
decoration: InputDecoration(
border: OutlineInputBorder(borderRadius: BorderRadius.circular(10)),
),
),
Container(height: 15),
const Divider(),
Container(height: 15),
Align(alignment: Alignment.center, child:
SizedBox(
width: 120,
height: 40,
child: ElevatedButton( //決定ボタン
onPressed: () {
state.widget.title = textController.text;
Navigator.pop(context);
},
child: const Text('決定')
),
),
),
],
);
},
);
},
);
}
void showAlert( String msg, BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return AlertDialog(
content: Text(msg),
actions: [
TextButton(
onPressed: () {
Navigator.pop(context, 'OK');
},
child: const Text('OK'))
],);
} );
}
Future<dynamic> relationSelectDialog(BuildContext context, CanvasZoneState state){
var isSelected = <bool>[false, false, false, false];
GlobalKey toggleButtonsKey = GlobalKey();
GlobalKey dialogKey = GlobalKey();
final TextEditingController textController = TextEditingController();
IdeasRelation? targetRelation = state.ref.read(selectedRelation);
if(targetRelation != null) {
if(targetRelation.fromShape == RelationShape.dot && targetRelation.toShape == RelationShape.dot) {
isSelected[0] = true;
} else if(targetRelation.fromShape == RelationShape.dot && targetRelation.toShape == RelationShape.arrow) {
// originとrelationの左右の位置によって、反映対象を変える
if (targetRelation.fromIdea.parentLane.indexX <= targetRelation.toIdea.parentLane.indexX) {
isSelected[1] = true;
} else {
isSelected[2] = true;
}
} else if(targetRelation.fromShape == RelationShape.arrow && targetRelation.toShape == RelationShape.dot) {
if (targetRelation.fromIdea.parentLane.indexX <= targetRelation.toIdea.parentLane.indexX) {
isSelected[2] = true;
} else {
isSelected[1] = true;
}
} else if(targetRelation.fromShape == RelationShape.arrow && targetRelation.toShape == RelationShape.arrow) {
isSelected[3] = true;
}
textController.text = targetRelation.note;
}
return showDialog(
context: context,
builder: (context) {
return StatefulBuilder(
builder: (context, setState) {
return SimpleDialog(
key: dialogKey,
contentPadding: const EdgeInsets.all(26),
children: [
Align( // 閉じるボタン
alignment: Alignment.centerRight,
child: Tooltip(
message: MaterialLocalizations
.of(context)
.closeButtonTooltip,
child: GestureDetector(
onTap: () => Navigator.pop(context),
child: const Icon(Icons.close, size: 30),
),
),
),
const Text('矢印の種類'),
Container(height: 10,),
ToggleButtons(
key: toggleButtonsKey,
direction: Axis.vertical,
borderColor: Colors.white,
selectedColor: Colors.lightBlueAccent,
selectedBorderColor: Colors.white,
isSelected: isSelected,
onPressed: (index) {
// The button that is tapped is set to true, and the others to false.
setState(() {
for (int i = 0; i < isSelected.length; i++) {
isSelected[i] = i == index;
}
});
},
children: [
SizedBox(
height: 40,
width: 180,
child: Stack(
children: drawRelationLineAt(state, 30,20,150,20, RelationShape.dot, RelationShape.dot, null),
),
),
SizedBox(
height: 40,
width: 180,
child: Stack(
children: drawRelationLineAt(state, 30,20,150,20, RelationShape.dot, RelationShape.arrow, null),
),
),
SizedBox(
height: 40,
width: 180,
child: Stack(
children: drawRelationLineAt(state, 30,20,150,20, RelationShape.arrow, RelationShape.dot, null),
),
),
SizedBox(
height: 40,
width: 180,
child: Stack(
children: drawRelationLineAt(state, 30,20,150,20, RelationShape.arrow, RelationShape.arrow, null),
),
),
],
),
Container(height: 10),
const Text('線の説明'),
TextField(
controller: textController,
decoration: InputDecoration(
border: OutlineInputBorder(borderRadius: BorderRadius.circular(10)),
),
),
Container(height: 15),
const Divider(),
Container(height: 15),
Align(alignment: Alignment.center, child:
SizedBox(
width: 120,
height: 40,
child: ElevatedButton( //決定ボタン
onPressed: () {
if (isSelected[0]) {
targetRelation!.fromShape = RelationShape.dot;
targetRelation.toShape = RelationShape.dot;
} else if (isSelected[1]) {
// originとrelationの左右の位置によって、反映対象を変える
if (targetRelation!.fromIdea.parentLane.indexX <= targetRelation.toIdea.parentLane.indexX) {
targetRelation.fromShape = RelationShape.dot;
targetRelation.toShape = RelationShape.arrow;
} else {
targetRelation.fromShape = RelationShape.arrow;
targetRelation.toShape = RelationShape.dot;
}
} else if (isSelected[2]) {
// originとrelationの左右の位置によって、反映対象を変える
if (targetRelation!.fromIdea.parentLane.indexX <= targetRelation.toIdea.parentLane.indexX) {
targetRelation.fromShape = RelationShape.arrow;
targetRelation.toShape = RelationShape.dot;
} else {
targetRelation.fromShape = RelationShape.dot;
targetRelation.toShape = RelationShape.arrow;
}
} else if (isSelected[3]) {
targetRelation!.fromShape = RelationShape.arrow;
targetRelation.toShape = RelationShape.arrow;
}
targetRelation!.note = textController.text;
Navigator.pop(context);
},
child: const Text('決定')
),
),
),
Container(height: 15),
Align(alignment: Alignment.bottomCenter, child:
Consumer(
builder: (context, ref, child) {
return TextButton( //線の削除ボタン
style: ButtonStyle(
foregroundColor: MaterialStateProperty.all<Color>(Colors.redAccent),
),
onPressed: () async {
// 確認ダイアログを表示
var result = await showDialog<bool>(
context: context,
barrierDismissible: false,
builder: (BuildContext context) {
return AlertDialog(
title: const Text('確認'),
content: const Text('線を削除してよろしいですか?'),
actions: <Widget>[
TextButton(
child: const Text('Cancel'),
onPressed: () => Navigator.of(context).pop(false),
),
TextButton(
child: const Text('OK'),
onPressed: () => Navigator.of(context).pop(true),
),
],
);
},
);
if (result == true) {
//リレーション削除の実行
removeRelation( ref.watch(ideasRelationList.notifier).state , targetRelation! );
Navigator.pop(context);
}
},
child: const Text('線の削除')
);
}
),
),
],
);
},
);
},
);
}
Size _getTextSize(String text, TextStyle style) {
final TextPainter textPainter = TextPainter(
text: TextSpan(text: text, style: style), maxLines: 1, textDirection: TextDirection.ltr)
..layout(minWidth: 0, maxWidth: double.infinity);
return textPainter.size;
}
// 指定した座標を元にリレーションの線と文字を描画
List <Widget> drawRelationLineAt(CanvasZoneState state, double startX, double startY, double endX, double endY, RelationShape startShape, RelationShape endShape, IdeasRelation? ideasRelation) {
List <Widget> list = [];
final double dotSize = state.ref.watch(relationDotSize);
final double arrowSize = state.ref.watch(relationArrowSize);
double rotateAdjustX = 0;
double rotateAdjustY = 0;
double rotateAdjustXForTransparent = 0;
double rotateAdjustYForTransparent = 0;
double scrollDeltaX = 0;//state.ref.watch(canvasScrollDeltaX);
bool hoverFlg = false;
double lineWidthDiv2 = 1;
void focusEnter() {
state.ref.watch(relationHoverFlg.notifier).state = true;
state.ref.watch(selectedRelation.notifier).state = ideasRelation;
}
void focusExit() {
state.ref.watch(relationHoverFlg.notifier).state = false;
state.ref.watch(selectedRelation.notifier).state = null;
}
if(ideasRelation != null) {
if ( state.ref.watch(relationHoverFlg) && state.ref.read(selectedRelation) == ideasRelation ) {
hoverFlg = true;
}
}
// 指定座標にドットを描画
Widget makeDotAt(double dx, double dy) {
return Positioned(
top: dy - (dotSize / 2),
left: dx - (dotSize / 2) + scrollDeltaX,
width: dotSize,
height: dotSize,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: Container(
decoration: BoxDecoration(
color: hoverFlg ? Colors.lightBlueAccent : Colors.blue,
shape: BoxShape.circle,
),
),
),
);
}
/* ドットと三角の描画 */
if(startShape == RelationShape.dot) {
list.add( //ドットの描画 Origin
makeDotAt( startX, startY )
);
} else {
list.add( //三角の描画
Positioned(
top: startY - (arrowSize / 2),
left: startX - (arrowSize / 2) + scrollDeltaX,
child: Transform.rotate(
angle: (startX == endX) /*レーンが同じ場合*/ ? 0 : getRadian(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY),
alignment: Alignment.center,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: CustomPaint(
painter: TrianglePainterL(hoverFlg ? Colors.lightBlueAccent : Colors.blue),
child: SizedBox(
width: arrowSize,
height: arrowSize,
),
),
),
),
),
);
}
if(endShape == RelationShape.dot) {
list.add( //ドットの描画 Relation
makeDotAt( endX, endY )
);
} else {
list.add( //三角の描画
Positioned(
top: endY - (arrowSize / 2),
left: endX - (arrowSize / 2) + scrollDeltaX,
child: Transform.rotate(
angle: (startX == endX) /*レーンが同じ場合*/ ? (180 * pi / 180) : getRadian(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY),
alignment: Alignment.center,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: CustomPaint(
painter: TrianglePainterR(hoverFlg ? Colors.lightBlueAccent : Colors.blue),
child: SizedBox(
width: arrowSize,
height: arrowSize,
),
),
),
),
),
);
}
double lineTop=0,lineLeft=0,lineHeight=0,lineWidth=0;
/* 線の描画 */
if (startX != endX) {
/*レーンが違う場合*/
if ( (endY - startY) > 30) { //右側の点が下側。回転すると、線の太さだけずれて見えてしまうので、それを調整する
rotateAdjustX = 1;
rotateAdjustY = 1;
rotateAdjustXForTransparent = 2;
rotateAdjustYForTransparent = 0;
} else if ( (endY - startY) < -30) { //右側の点が上側。回転すると、線の太さだけずれて見えてしまうので、それを調整する
rotateAdjustX = -1;
rotateAdjustY = 0;
rotateAdjustXForTransparent = -2;
rotateAdjustYForTransparent = 0;
}
lineTop = startY - lineWidthDiv2 + rotateAdjustY;
lineLeft = startX + lineWidthDiv2 + scrollDeltaX + rotateAdjustX;
lineWidth = getDistance(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY) - 2;
lineHeight= 2;
list.add( //線の描画
Stack(
children:[
Positioned( //実際の線の描画
top: lineTop,
left: lineLeft,
child: Transform.rotate(
angle: getRadian(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY),
alignment: Alignment.topLeft,
origin: const Offset( -0.5, -0.5 ),
child: Container(
width: lineWidth,
height: lineHeight,
decoration: BoxDecoration(
color: hoverFlg ? Colors.lightBlueAccent : Colors.blue,
),
),
),
),
Positioned( //当たり判定用の透明な線
top: startY - lineWidthDiv2 + rotateAdjustY + rotateAdjustYForTransparent - 3/*線の太さ分を調整*/,
left: startX + lineWidthDiv2 + scrollDeltaX + rotateAdjustXForTransparent + rotateAdjustX - 1,
child: Transform.rotate(
angle: getRadian(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY),
alignment: Alignment.topLeft,
// origin: Offset( state.ref.watch(relationDotSize)/2 , state.ref.watch(relationDotSize)/2 ),
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: Container(
width: getDistance(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY),
height: 8,
decoration: const BoxDecoration( color: relationColorTransparent ),
),
),
),
),
]
),
);
} else {
/*レーンが同じ場合*/
double horizontalMargin = ideaHorizontalMargin - 2;
lineTop = startY - lineWidthDiv2;
lineLeft = startX + scrollDeltaX + horizontalMargin;
lineWidth = 2;
lineHeight= endY- startY + (lineWidthDiv2*2) /*線の太さ分を調整*/;
list.add( //線の描画
Stack(
children:[
Positioned( //実際の線の描画(縦)
top: lineTop,
left: lineLeft,
child: Container(
width: 2,
height: lineHeight,
decoration: BoxDecoration(
color: hoverFlg ? Colors.lightBlueAccent : Colors.blue,
),
),
),
Positioned( //実際の線の描画(横1)
top: startY - lineWidthDiv2,
left: startX + lineWidthDiv2 + scrollDeltaX,
child: Container(
width: horizontalMargin,
height: 2,
decoration: BoxDecoration(
color: hoverFlg ? Colors.lightBlueAccent : Colors.blue,
),
),
),
Positioned( //実際の線の描画(横2)
top: endY - lineWidthDiv2,
left: endX + lineWidthDiv2 + scrollDeltaX,
child: Container(
width: horizontalMargin,
height: 2,
decoration: BoxDecoration(
color: hoverFlg ? Colors.lightBlueAccent : Colors.blue,
),
),
),
Positioned( //当たり判定用の透明な線(縦)
top: startY - lineWidthDiv2 - 2 /*線の太さ分を調整*/,
left: startX + scrollDeltaX + horizontalMargin - 2,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: Container(
width: 2 + 4,
height: endY - startY + (lineWidthDiv2*2) /*線の太さ分を調整*/ + 4,
decoration: const BoxDecoration( color: relationColorTransparent ),
),
),
),
Positioned( //当たり判定用の透明な線(横1)
top: startY - lineWidthDiv2 - 2 /*線の太さ分を調整*/,
left: startX + lineWidthDiv2 + scrollDeltaX,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: Container(
width: horizontalMargin,
height: 2 + 4,
decoration: const BoxDecoration( color: relationColorTransparent ),
),
),
),
Positioned( //当たり判定用の透明な線(横2)
top: endY - lineWidthDiv2 - 2 /*線の太さ分を調整*/,
left: endX + lineWidthDiv2 + scrollDeltaX,
child: MouseRegion(
onEnter: (_) => focusEnter(),
onExit: (_) => focusExit(),
child: Container(
width: horizontalMargin,
height: 2 + 4,
decoration: const BoxDecoration( color: relationColorTransparent ),
),
),
),
]
),
);
}
String note = '';
if(ideasRelation != null) {
note = ideasRelation.note;
}
/* 文字の描画 */
if ( note != '' ) {
/* 線の描画 */
if (startX != endX) {
/*レーンが違う場合*/
double radian = getRadian(startX + scrollDeltaX, startY, endX + scrollDeltaX, endY);
// radianのイメージ
// -90° = (-90* pi / 180) =-1.5707963267948966
// -45° = (-45* pi / 180) =-0.7853981633974483
// 0° = ( 0 * pi / 180) = 0
// 45° = (45 * pi / 180) = 0.7853981633974483
// 90° = (90 * pi / 180) = 1.5707963267948966
// 180° = pi = 3.14
// 手入力での調整結果
// -90° left: - 18, top: - 0,
// -75° left: - 15, top: - 8,
// -45° left: - 12, top: - 12,
// -30° left: - 5, top: - 15,
// 0° left: - 0, top: - 18,
//
// Offset(13*radian, -19 + (5*radian.abs())),
// -90° left: -20.4, top: - 19+7.8= -11.2
// -45° left: -10.2, top: - 19+3.9= -15.1
// 0° left: 0, top: - 19,
// 45° left: 10.2, top: - 19+3.9= -15.1
// 90° left: 20.4, top: - 19+7.8= -11.2
//
//print("$radian (-45 * pi / 180):${(-45 * pi / 180)} (90 * pi / 180):${(90 * pi / 180)} (180 * pi / 180):${(180 * pi / 180)}");
list.add( //文字の描画
Stack(
children: [
Positioned( //文字の描画
top: lineTop,
left: lineLeft,
width: lineWidth,
child: Align(
alignment: Alignment.center,
child: Transform.translate(
offset: Offset(13 * radian, -19 + (4 * radian.abs())),
child: Transform.rotate(
angle: radian,
alignment: Alignment.topLeft,
origin: const Offset(-0.5, -0.5),
child: Container(
color: Colors.transparent,
alignment: Alignment.center,
child: Text(note, style: const TextStyle(color: Colors.blue),),
),
),
),
),
),
]
),
);
} else {
/*レーンが同じ場合*/
double radian = getRadian(lineLeft, lineTop, lineLeft, lineTop+lineHeight);
Size textSize = _getTextSize(note, const TextStyle(color: Colors.blue));
list.add( //文字の描画
Stack(
children: [
Positioned( //文字の描画
top: lineTop,
left: lineLeft,
child: Align(
alignment: Alignment.center,
child: Transform.translate(
offset: Offset(textSize.height + 4, (lineHeight/2) - (textSize.width/2) ),
child: Transform.rotate(
angle: radian,
alignment: Alignment.topLeft,
origin: const Offset(-0.5, -0.5),
child: Container(
color: Colors.transparent,
alignment: Alignment.center,
child: Text(note, style: const TextStyle(color: Colors.blue),),
),
),
),
),
),
]
),
);
}
}
//デバッグ用
if(relationDebugPaint) {
list.add(
Positioned( //デバッグ用
top: startY,
left: startX + scrollDeltaX,
width: 1,
height: 1,
child: Container(
decoration: const BoxDecoration(
color: Colors.red,
shape: BoxShape.circle,
),
),
),
);
list.add(
Positioned( //デバッグ用
top: startY - lineWidthDiv2 + rotateAdjustY,
left: startX + lineWidthDiv2 + scrollDeltaX,
width: 1,
height: 1,
child: Container(
decoration: const BoxDecoration(
color: Colors.orange,
shape: BoxShape.circle,
),
),
),
);
}
return list;
}
// relationを元にリレーション描画ウィジェットを作成
List<Widget> buildRelationLineWidget(CanvasZoneState state, IdeasRelation relationSet) {
Idea startIdea, endIdea;
RelationShape startShape, endShape;
double startX, startY, endY, endX;
final double laneWidth = state.ref.watch(eachLaneWidth);
final double ideaHeight = GlobalConst.eachIdeaHeight;
if (relationSet.fromIdea.parentLane.indexX != relationSet.toIdea.parentLane.indexX ) {
// leftを必ずstartIdeaとしてしまう。左右逆のパターンもロジックを記述すると、回転の計算などが面倒になったため
if (relationSet.fromIdea.parentLane.indexX <= relationSet.toIdea.parentLane.indexX) {
startIdea = relationSet.fromIdea;
startShape = relationSet.fromShape;
endIdea = relationSet.toIdea;
endShape = relationSet.toShape;
} else {
startIdea = relationSet.toIdea;
startShape = relationSet.toShape;
endIdea = relationSet.fromIdea;
endShape = relationSet.fromShape;
}
// 描画位置から求めると、パン・スクロールしたときにどんどんずれていく
// RenderBox originIdeaTextFieldBox = origin.textFieldKey.currentContext?.findRenderObject() as RenderBox;
startY = (ideaHeight * startIdea.index) + (ideaHeight/2) - 0.5/*調整用。これが無いとずれて見える*/ ;
startX = (startIdea.parentLane.indexX + 1) * laneWidth - ideaHorizontalMargin - 0.5/*調整用。これが無いとずれて見える*/;
endY = (ideaHeight * endIdea.index) + (ideaHeight/2) - 0.5/*調整用。これが無いとずれて見える*/;
endX = (laneWidth * endIdea.parentLane.indexX) + ideaHorizontalMargin + ideaLeftSpace + (GlobalConst.ideaIndentWidth * endIdea.indentLevel) + 0.5/*調整用。これが無いとずれて見える*/;
/* 見やすくするためのずらし調整(インデント) */
if (startIdea.parentLane.indexX < endIdea.parentLane.indexX &&
endIdea.indentLevel >= 1) { //Relatedが右側にあり、インデントされている場合
endY += 3; //Related側のインデントの線と重なるのを避けるために少しずらす
}
/* 見やすくするためのずらし調整(他の矢印やドットとの重なり:右側) */
if ( (endY - startY).abs() > 10) { // 線に角度が付いている場合
bool avoidFlg = false;
//対象のアイディアに、他にリレーションが付いているか探す
if(endIdea.relatedIdeasFrom.length >= 2) {
avoidFlg = true;
}
if (avoidFlg) {
if (startY < endY - 10) { // 矢印が斜めの場合
endY -= 6; //Related側の矢印と重なるのを避けるために少しずらす
} else if (startY > endY) {
endY += 6;
}
}
/* 見やすくするためのずらし調整(他の矢印やドットとの重なり:右側) */
avoidFlg = false;
//対象のアイディアに、他にリレーションが付いているか探す
if(startIdea.relatedIdeasTo.length >= 2) {
avoidFlg = true;
}
if (avoidFlg) {
if (startY < endY - 10) { // 矢印が斜めの場合
startY += 6; //Related側の矢印と重なるのを避けるために少しずらす
} else if (startY > endY) {
startY -= 6;
}
}
}
} else{
/* 同じレーン同士の場合 */
// 計算を簡単にするため、上を必ずstartIdeaとしてしまう
if (relationSet.fromIdea.index <= relationSet.toIdea.index) {
startIdea = relationSet.fromIdea;
startShape = relationSet.fromShape;
endIdea = relationSet.toIdea;
endShape = relationSet.toShape;
} else {
startIdea = relationSet.toIdea;
startShape = relationSet.toShape;
endIdea = relationSet.fromIdea;
endShape = relationSet.fromShape;
}
startY = (ideaHeight * startIdea.index) + (ideaHeight/2) - 0.5/*調整用。これが無いとずれて見える*/ ;
startX = (startIdea.parentLane.indexX + 1) * laneWidth - ideaHorizontalMargin - 0.5/*調整用。これが無いとずれて見える*/;
endY = (ideaHeight * endIdea.index) + (ideaHeight/2) - 0.5/*調整用。これが無いとずれて見える*/;
endX = startX;
/* 見やすくするためのずらし調整(他の矢印やドットとの重なり:右側) */
bool avoidFlg = false;
//対象のアイディアに、他にリレーションが付いているか探す
if(startIdea.relatedIdeasTo.length >= 2) {
avoidFlg = true;
}
if (avoidFlg) {
if (startY < endY - 10) { // 矢印が斜めの場合
startY += 6; //Related側の矢印と重なるのを避けるために少しずらす
} else if (startY > endY) {
startY -= 6;
}
}
}
if(relationDebugPaint) {
List<Widget> result = drawRelationLineAt(state, startX,startY,endX,endY, startShape,endShape, relationSet) ;
//座標に関するデバッグ用 originの表示
result.add(
Stack(children: [
Positioned( //デバッグ用 originの表示
top: startIdea == relationSet.fromIdea ? startY : endY,
left: startIdea == relationSet.fromIdea ? startX + state.ref.watch(canvasScrollDeltaX) : endX + state.ref.watch(canvasScrollDeltaX),
child: Container(
width: 3,
height: 3,
decoration: const BoxDecoration(color: Colors.deepPurpleAccent,),
),
),
])
);
//デバッグ用 dotの中心線
result.add(
Stack(children: [
Positioned( //デバッグ用
top: endY,
left: endX + state.ref.watch(canvasScrollDeltaX),
child: Container(
width: 500,
height: 0.5,
decoration: const BoxDecoration(color: Colors.red,),
),
),
])
);
return result;
} else {
return drawRelationLineAt(state, startX,startY,endX,endY, startShape,endShape, relationSet) ;
}
}
List <Widget> buildIdeasAndRelations(CanvasZoneState state) {
List<Widget> list = [];
final double laneWidth = state.ref.watch(eachLaneWidth);
// print("buildIdeasAndRelations state.ref.watch(allHorizontalLanesHeights):${state.ref.watch(allHorizontalLanesHeights)} state.ref.watch(lanesBodyCanvasHeight)${state.ref.watch(lanesBodyCanvasHeight)}");
double getWidthOfAllLanes() {
int maxLength = 0;
state.horizontalLanes.forEach((element) {
if ( element.childVLaneBodies.length > maxLength ) {
maxLength = element.childVLaneBodies.length;
}
});
return maxLength * state.ref.watch(eachLaneWidth);
}
/* Ideas */
for (int i=0; i<state.horizontalLanes.length; i++){
// print("state.horizontalLanes[$i].viewHeight:${state.horizontalLanes[i].viewHeight}");
var aHorizontalLane = state.horizontalLanes[i];
if(aHorizontalLane.index != 0) {
list.add(
Positioned( //区切り線
top: state.horizontalLanes[aHorizontalLane.index-1].viewTop+ state.horizontalLanes[aHorizontalLane.index-1].viewHeight,
left: 0,
child: Container (
width: MediaQuery.of(state.context).size.width,//getWidthOfAllLanes(),
height: GlobalConst.horizontalLaneSplitterHeight,
color: Colors.transparent,
child: Align(
alignment: Alignment.centerLeft,
child: Container (
height: 1.0,
color: Colors.blue,
),
)
),
),
);
}
list.add(
Positioned( //横レーンの1つの配置
top: aHorizontalLane.viewTop,
left: 0,
width: laneWidth * aHorizontalLane.childVLaneBodies.length,
height: aHorizontalLane.viewHeight,//state.ref.watch(lanesBodyCanvasHeight),
child: ListView.builder( //横レーン内の、縦1レーンごとの箱の生成
scrollDirection: Axis.horizontal,
itemCount: aHorizontalLane.childVLaneBodies.length,
itemBuilder: (context, index) {
if((aHorizontalLane.childVLaneBodies[index].requiredHeight <= aHorizontalLane.viewHeight)) {
// 横レーンのビュー高さよりも縦1レーンの描画が小さい場合
return Container(
width: laneWidth,
child: aHorizontalLane.childVLaneBodies[index],
);
} else {
// 横レーンのビュー高さより縦1レーンの高さが大きい場合
return Container( //ビューポート用
width: laneWidth,
height: aHorizontalLane.viewHeight,
child: Scrollbar(
controller: aHorizontalLane.childVLaneBodies[index].scrollController,
thumbVisibility: true,
child: SingleChildScrollView(
controller: aHorizontalLane.childVLaneBodies[index].scrollController,
primary: false,
child: Container(
width: laneWidth,
height: aHorizontalLane.childVLaneBodies[index].requiredHeight,
child: SizedBox(
width: laneWidth,
child: aHorizontalLane.childVLaneBodies[index],
),
),
),
),
);
}
},
),
),
);
}
if (state == null) return list;
if (state.firstVerticalLanes.isEmpty) return list;
if (state.firstVerticalLanes[0].ideas.isEmpty) return list;
/* Relation */
IdeasRelation? lazyBuildTarget;
for (IdeasRelation relationSet in state.ref.watch(ideasRelationList)) {
if ( state.ref.watch(relationHoverFlg) && state.ref.read(selectedRelation) == relationSet ) {
//アクティブな線は、最後に描画することで手前に持ってくる(そうしないと他の線に隠れてしまう)
lazyBuildTarget = relationSet;
} else {
list.addAll( buildRelationLineWidget(state, relationSet) );
}
}
if(lazyBuildTarget != null) {
list.addAll( buildRelationLineWidget(state, lazyBuildTarget) );
}
/*
//デバッグ用
list.add(
Stack(children: [
Positioned( //デバッグ用
top: 0,
left: state.ref.watch(eachLaneWidth) - ideaHorizontalMargin - 50 + state.ref.watch(canvasScrollDeltaX),
child: Container(
width: 500,
height: 1,
decoration: BoxDecoration(color: Colors.red,),
),
),
Positioned( //デバッグ用
top: (ideaHeight / 2),
left: state.ref.watch(eachLaneWidth) - ideaHorizontalMargin - 50 + state.ref.watch(canvasScrollDeltaX),
child: Container(
width: 1000,
height: 0.5,
decoration: BoxDecoration(color: Colors.green,),
),
),
],
),
);
*/
return list;
}
void indentLinePaint(Canvas canvas,Size size,BuildContext context, int index, int indentLevel, double textFieldHeight, double indentWidth) {
// インデントの線を描画する
final paint = Paint()
..color = Colors.grey
..strokeWidth = 1;
var leftX = indentWidth / 2;
// 一行上のIdeaのindentLevelを取得する
int upperIndentLevel = 0;
int upperIndex = index - 1;
//描画:Canvasの起点は左側センター
if (indentLevel > 0 && index > 0 /*一番上の時はツリーを描画しない*/) {
//一番上の親が居ない場合の特別対応:自分の上のどこかに、indentLevelが小さい親がいるかチェック
bool cancelFlg = false; //描画しない特別な場合用
var i = index - 1;
while (i >= 0) {
if ((LaneBodyInheritedWidget.of(context)
.ideas[i].indentLevel) <
indentLevel) {
break;
}
i--;
}
if (i == -1) cancelFlg = true;
if (!cancelFlg) {
//縦の線:左側の基準
leftX = leftX + (indentWidth * (indentLevel - 1));
//縦の線:自分の領域内で上に引く
double startY = 0 - (textFieldHeight / 2) - (ideaVerticalMargin * 2);
//縦の線:自分の領域外でどのくらい上まで引くかを計算
upperIndentLevel = LaneBodyInheritedWidget.of(context)
.ideas[upperIndex].indentLevel;
if (indentLevel <= upperIndentLevel) {
while (indentLevel < upperIndentLevel && upperIndex >= 0) {
startY -= (textFieldHeight + ideaVerticalMargin * 2);
upperIndex--;
upperIndentLevel = LaneBodyInheritedWidget.of(context)
.ideas[upperIndex].indentLevel;
}
if (indentLevel <= upperIndentLevel) {
startY -= textFieldHeight / 2;
}
}
//縦の線
canvas.drawLine(Offset(leftX, 0), Offset(leftX, startY), paint);
//横の線
canvas.drawLine(Offset(leftX, size.height),Offset(indentWidth * indentLevel, size.height), paint);
}
}
}
void findAndRepaintAffectedIdeas(BuildContext context, int myIndex, int myIndentLevel, int startIndex, int endIndex){
// Find the start index
for (int i = myIndex - 1; i >= 0; i--) {
if (LaneBodyInheritedWidget.of(context)
.ideas[i].indentLevel == myIndentLevel - 1) {
startIndex = i;
break;
}
}
// Find the end index
for (int i = myIndex + 1;
i < LaneBodyInheritedWidget.of(context).ideas.length;
i++) {
if ((LaneBodyInheritedWidget.of(context)
.ideas[i].indentLevel) >= myIndentLevel) {
endIndex = i;
break;
}
}
CanvasZoneInheritedWidget.of(context).setState(() {}); //Relationの線を描画
}
Future<String> get _defaultFileName async {
final directory = await getApplicationDocumentsDirectory();
return '${directory.path}/default.json';
}
Future<void> saveDataToFile({String? fileName, required CanvasZoneState? state}) async {
List<Map<String, dynamic>> horizontalLanesJson = [];
fileName ??= await _defaultFileName;
if(state == null) return;
for (var horizontalLane in state.horizontalLanes ) {
List<Map<String, dynamic>> verticalLanesJson = [];
for (var verticalLane in horizontalLane.childVLaneBodies) {
List<Map<String, dynamic>> ideasJson = [];
for (var idea in verticalLane.ideas) {
ideasJson.add({
'index': idea.index,
'indentLevel': idea.indentLevel,
'content': idea.key.currentState?.textController.text ?? '',
});
}
if(horizontalLane.index == 0) {
verticalLanesJson.add({
'index': verticalLane.indexX,
'verticalTitle': state.verticalLaneHeaders[verticalLane.indexX].key.currentState?.titleController.text ?? '',
'ideas': ideasJson,
});
} else {
verticalLanesJson.add({
'index': verticalLane.indexX,
'ideas': ideasJson,
});
}
}
horizontalLanesJson.add({
'index': horizontalLane.index,
'horizontalTitle': horizontalLane.header.title,
'horizontalViewHeight': horizontalLane.viewHeight,
'verticalLanes': verticalLanesJson,
});
}
List<Map<String, dynamic>> ideasRelationJson = [];
state.ref.read(ideasRelationList).forEach((relationSet) {
ideasRelationJson.add({
'from_lane_index': relationSet.fromIdea.parentLane.indexX,
'from_idea_index': relationSet.fromIdea.index,
'from_shape': relationSet.fromShape == RelationShape.dot ? 'dot' : 'arrow',
'to_lane_index': relationSet.toIdea.parentLane.indexX,
'to_idea_index': relationSet.toIdea.index,
'to_shape': relationSet.toShape == RelationShape.dot ? 'dot' : 'arrow',
'note': relationSet.note,
});
});
String jsonString = jsonEncode({'horizontalLanes': horizontalLanesJson, 'ideasRelation': ideasRelationJson});
await File(fileName).writeAsString(jsonString);
}
Future<void> loadDataFromFile({String? fileName, required CanvasZoneState? state}) async {
fileName ??= await _defaultFileName;
final file = File(fileName);
if (await file.exists() == false) {
showAlert('No such files:{$fileName}', state!.context);
return;
}
String jsonString = await file.readAsString();
Map<String, dynamic> jsonMap = jsonDecode(jsonString);
if(state == null) return;
state.clearCurrentData();
List<VerticalLaneHeader> loadedVerticalLaneHeaders = [];
for (var horizontalLaneJson in jsonMap['horizontalLanes']) {
var newHorizontalLane = HorizontalLane(
horizontalLaneJson['index'] ?? 0,
horizontalLaneJson['horizontalTitle'] ?? ''
);
newHorizontalLane.viewHeight = horizontalLaneJson['viewHeight'] ?? GlobalConst.horizontalLaneDefaultViewHeight;
List<LaneBody> newVerticalLanes = [];
for (var verticalLaneJson in horizontalLaneJson['verticalLanes']) {
List<Idea> loadedIdeas = [];
var newLane = LaneBody(
indexX: verticalLaneJson['index'] ?? 0,
indexY: newHorizontalLane.index,
title: verticalLaneJson['verticalTitle'] ?? '',
ideas: loadedIdeas,
);
if(newHorizontalLane.index == 0){
var newLaneHeader = VerticalLaneHeader(index: verticalLaneJson['index'] ?? 0, relatedLane: newLane);
newLaneHeader.title = verticalLaneJson['verticalTitle'] ?? '';
loadedVerticalLaneHeaders.add(newLaneHeader);
}
for (var ideaJson in verticalLaneJson['ideas']) {
loadedIdeas.add(Idea(
index: ideaJson['index'],
indentLevel: ideaJson['indentLevel'],
parentLane: newLane,
));
}
newLane.updateRequiredHeight();
newVerticalLanes.add(newLane);
for (int i = 0; i < newVerticalLanes.length; i++) {
for (int j = 0; j < newVerticalLanes[i].ideas.length; j++) {
newVerticalLanes[i].ideas[j].content = jsonMap['horizontalLanes'][newHorizontalLane.index]['verticalLanes'][i]['ideas'][j]['content'];
}
}
}
newHorizontalLane.childVLaneBodies = newVerticalLanes;
state.horizontalLanes.add(newHorizontalLane);
}
state.firstVerticalLanes = state.horizontalLanes[0].childVLaneBodies;
// state.horizontalLanes[0].childLaneBodies = loadedVerticalLanes;
state.verticalLaneHeaders = loadedVerticalLaneHeaders;
if (jsonMap['ideasRelation'] != null) {
for (var ideaRelationJson in jsonMap['ideasRelation']) {
var fromLaneIndex = ideaRelationJson['from_lane_index'];
var fromIdeaIndex = ideaRelationJson['from_idea_index'];
var toLaneIndex = ideaRelationJson['to_lane_index'];
var toIdeaIndex = ideaRelationJson['to_idea_index'];
IdeasRelation relationSet = IdeasRelation(
fromIdea: state.firstVerticalLanes[fromLaneIndex].ideas[fromIdeaIndex], toIdea: state.firstVerticalLanes[toLaneIndex].ideas[toIdeaIndex]);
if(ideaRelationJson['from_shape'] == 'arrow') {
relationSet.fromShape = RelationShape.arrow;
}
if(ideaRelationJson['to_shape'] == 'arrow') {
relationSet.toShape = RelationShape.arrow;
}
relationSet.note = ideaRelationJson['note'];
state.ref.watch(ideasRelationList.notifier).state.add(relationSet);
state.firstVerticalLanes[fromLaneIndex].ideas[fromIdeaIndex].relatedIdeasTo.add(state.firstVerticalLanes[toLaneIndex].ideas[toIdeaIndex]);
state.firstVerticalLanes[toLaneIndex].ideas[toIdeaIndex].relatedIdeasFrom.add(state.firstVerticalLanes[fromLaneIndex].ideas[fromIdeaIndex]);
}
}
}
class Tategaki extends StatelessWidget {
Tategaki(
this.text, {
this.style,
this.space = 12,
});
final String text;
final TextStyle? style;
final double space;
@override
Widget build(BuildContext context) {
final splitText = text.split("\n");
return Column(
textDirection: TextDirection.rtl,
children: [
for (var s in splitText) _textBox(s.runes),
],
);
}
Widget _textBox(Runes runes) {
return Wrap(
textDirection: TextDirection.rtl,
direction: Axis.vertical,
children: [
for (var rune in runes)
Row(
children: [
// SizedBox(width: space),
SizedBox(
width: 30,
height: 25,
child:
Align(
alignment: (String.fromCharCode(rune) == '、' || String.fromCharCode(rune) == '。') ? Alignment.topRight : Alignment.center,
child: _character(String.fromCharCode(rune)),
),
),
],
)
],
);
}
Widget _character(String char) {
if (VerticalRotated.map[char] != null) {
return Text(VerticalRotated.map[char]!, style: style);
} else {
return Text(char, style: style);
}
}
}
class VerticalRotated {
static const map = {
' ': ' ',
'↑': '→',
'↓': '←',
'←': '↑',
'→': '↓',
'。': '︒',
'、': '︑',
'ー': '丨',
'─': '丨',
'-': '丨',
'ー': '丨',
'_': '丨 ',
'−': '丨',
'-': '丨',
'—': '丨',
'〜': '丨',
'~': '丨',
'/': '\',
'…': '︙',
'‥': '︰',
'︙': '…',
':': '︓',
':': '︓',
';': '︔',
';': '︔',
'=': '॥',
'=': '॥',
'(': '︵',
'(': '︵',
')': '︶',
')': '︶',
'[': '﹇',
"[": '﹇',
']': '﹈',
']': '﹈',
'{': '︷',
'{': '︷',
'<': '︿',
'<': '︿',
'>': '﹀',
'>': '﹀',
'}': '︸',
'}': '︸',
'「': '﹁',
'」': '﹂',
'『': '﹃',
'』': '﹄',
'【': '︻',
'】': '︼',
'〖': '︗',
'〗': '︘',
'「': '﹁',
'」': '﹂',
',': '︐',
'、': '︑',
};
}
settings.dart については変更なし
※本記事は当時の記録をもとに作成し、必要に応じて加筆・補足しています
テキストベースの思考整理ツール「アイディア・レーン」最新版はこちら。
コメント